Python: Find the maximum profit in one transaction
Max Stock Profit (One Transaction)
The price of a given stock on each day is stored in an array.
Write a Python program to find the maximum profit in one transaction i.e., buy one and sell one share of the stock from the given price value of the said array. You cannot sell a stock before you buy one.
Input (Stock price of each day): [224, 236, 247, 258, 259, 225]
Output: 35
Explanation:
236 - 224 = 12
247 - 224 = 23
258 - 224 = 34
259 - 224 = 35
225 - 224 = 1
247 - 236 = 11
258 - 236 = 22
259 - 236 = 23
225 - 236 = -11
258 - 247 = 11
259 - 247 = 12
225 - 247 = -22
259 - 258 = 1
225 - 258 = -33
225 - 259 = -34
Sample Solution:
Python Code:
Sample Output:
35
Explanation:
Here is a breakdown of the above Python code:
- The function "max_profit()" calculates the maximum profit that can be obtained by buying and selling stocks.
- It initializes the maximum profit amount (max_profit_amt) to zero.
- It uses a nested loop to iterate through the stock prices.
- For each day (outer loop), it initializes the profit amount (profit_amt) to zero.
- It then iterates through the subsequent days (inner loop) to calculate potential profits.
- The profit is calculated by subtracting the buying price (stock_price[i]) from the selling price (stock_price[j]).
- If the calculated profit is greater than the current maximum profit (max_profit_amt), it updates the maximum profit.
- Finally, it returns the maximum profit amount.
Flowchart:
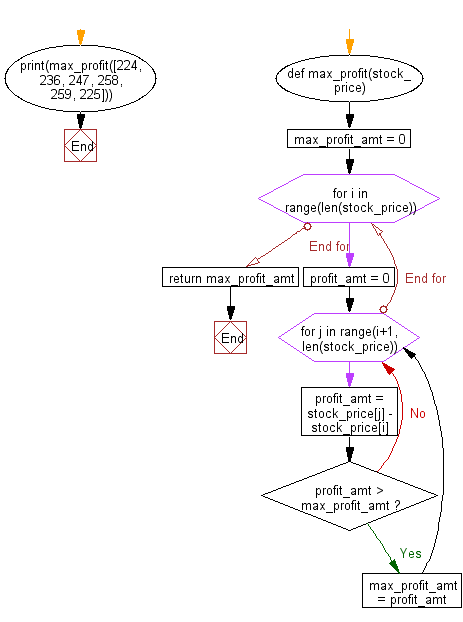
For more Practice: Solve these Related Problems:
- Write a Python program to find the maximum profit from a single stock transaction by tracking the minimum price.
- Write a Python program to compute the maximum profit from stock prices using dynamic programming.
- Write a Python program to determine the optimal buy and sell points in an array of stock prices for maximum gain.
- Write a Python program to calculate the maximum possible profit by iterating through the price list and updating the best profit dynamically.
Go to:
Previous: Write a Python program to find the starting and ending position of a given value in a given array of integers, sorted in ascending order.
Next: Write a Python program to print a given N by M matrix of numbers line by line in forward > backwards > forward >... order.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.