Python: Remove all instances of a given value from a given array of integers and find the length of the new array
Remove Value from Array
Write a Python program to remove all instances of a given value from a given array of integers and find the length of the newly created array.
Sample Solution:
Python Code:
# Define a function to remove elements equal to a given value from a list
def remove_element(array_nums, val):
# Initialize a variable to iterate through the list
i = 0
# Iterate through the list
while i < len(array_nums):
# Check if the current element is equal to the given value
if array_nums[i] == val:
# Remove the element if it matches the given value
array_nums.remove(array_nums[i])
else:
# Move to the next index if the elements do not match
i += 1
# Return the length of the modified list
return len(array_nums)
# Test the function with different lists and values, and print the results
print(remove_element([1, 2, 3, 4, 5, 6, 7, 5], 5))
print(remove_element([10, 10, 10, 10, 10], 10))
print(remove_element([10, 10, 10, 10, 10], 20))
print(remove_element([], 1))
Sample Output:
6 0 5 0
Explanation:
Here is a breakdown of the above Python code:
- The function "remove_element()" removes elements equal to a given value from a list.
- It initializes a variable 'i' to iterate through the list.
- It iterates through the list using a while loop.
- If the current element is equal to the given value, it removes that element from the list.
- If the elements do not match, it moves to the next index.
- The function returns the length of the modified list.
- Test the function with different lists and values, and print the results.
Visual Presentation:
Flowchart:
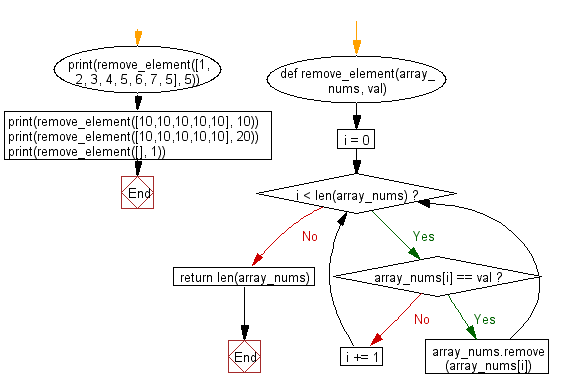
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to calculate the maximum profit from selling and buying values of stock. An array of numbers represent the stock prices in chronological order.
Next: Write a Python program to find the starting and ending position of a given value in a given array of integers, sorted in ascending order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics