Python: Check whether a given integer is a palindrome or not
Integer Palindrome Checker
Write a Python program to check whether a given integer is a palindrome or not.
Note: An integer is a palindrome when it reads the same backward as forward. Negative numbers are not palindromic.
Sample Solution:
Python Code:
# Define a function to check if a number is a palindrome
def is_Palindrome(n):
return str(n) == str(n)[::-1]
# Test cases
print(is_Palindrome(100)) # False
print(is_Palindrome(252)) # True
print(is_Palindrome(-838)) # False
Sample Output:
False True False
Explanation:
Here is a breakdown of the above Python code:
- The function "is_Palindrome()" takes an integer 'n' as input.
- It converts the integer to a string using str(n).
- The expression str(n)[::-1] reverses the string.
- The function returns 'True' if the original string is equal to its reversed version, indicating that the number is a palindrome.
- Test the function with three different inputs and print the results.
Visual Presentation:
Flowchart:
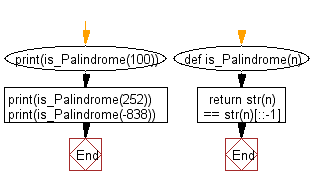
For more Practice: Solve these Related Problems:
- Write a Python program to check if an integer is a palindrome by converting it to a string and comparing it to its reverse.
- Write a Python program to determine if a number is palindromic without using string conversion.
- Write a Python program to verify integer palindromes by comparing digits using arithmetic operations.
- Write a Python program to check if a positive integer reads the same backwards and forwards by iterative digit extraction.
Go to:
Previous: Write a Python program to reverse only the vowels of a given string.
Next: Write a Python program to remove the duplicate elements of a given array of numbers such that each element appear only once and return the new length of the given array.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.