Python: Check whether the sum of any two numbers from the list is equal to k or not
Pair Sum to Target
Given a list of numbers and a number k, write a Python program to check whether the sum of any two numbers from the list is equal to k or not.
For example, given [1, 5, 11, 5] and k = 16, return true since 11 + 5 is 16.
Sample Solution:
Python Code:
# Function to check if there exists a pair in the list whose sum is equal to the given value 'k'
def check_sum(nums, k):
# Loop through each element in the list
for i in range(len(nums)):
# Nested loop to compare each element with subsequent elements
for j in range(i+1, len(nums)):
# Check if the sum of the current pair is equal to 'k'
if nums[i] + nums[j] == k:
return True # Return True if such a pair is found
return False # Return False if no such pair is found
# Test cases
print(check_sum([12, 5, 0, 5], 10))
print(check_sum([20, 20, 4, 5], 40))
print(check_sum([1, -1], 0))
print(check_sum([1, 1, 0], 0))
Sample Output:
True True True False
Explanation:
Here is a breakdown of the above Python code:
- Define a function named "check_sum()" that takes a list of numbers (nums) and a target sum (k) as input.
- Use a nested loop to iterate through each pair of elements in the list.
- Check if the sum of the current pair is equal to the target sum (k).
- If such a pair is found, return 'True'.
- If no such pair is found after checking all pairs, return 'False'.
- Test the function with different inputs to check its functionality.
Visual Presentation:
Flowchart:
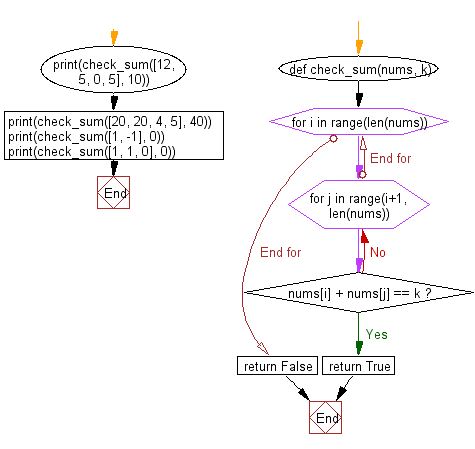
For more Practice: Solve these Related Problems:
- Write a Python program to determine if any two numbers in a list add up to a given target using a hash set.
- Write a Python program to check for the existence of a pair that sums to k using the two-pointer technique on a sorted list.
- Write a Python program to return all unique pairs from a list whose sum equals a target value.
- Write a Python program to verify if any two numbers in an unsorted array can be paired to produce a given sum without nested loops.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program which adds up columns and rows of given table.
Next: Write a Python program to find the longest word in set of words which is a subsequence of a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.