Python: Convert the string to a list and print all the words and their frequencies
Word Frequency Counter
Write a Python program that prints long text, converts it to a list, and prints all the words and the frequency of each word.
Sample Solution:
Python Code :
# Define a multiline string containing a passage about the United States Declaration of Independence.
string_words = '''United States Declaration of Independence
From Wikipedia, the free encyclopedia
... (omitting the rest for brevity) ...
... much scholarly inquiry.
The Declaration justified the independence of the United States by listing colonial grievances against
King George III, and by asserting certain natural and legal rights, including a right of revolution.
Having served its original purpose in announcing independence, references to the text of the
Declaration were few in the following years. Abraham Lincoln made it the centerpiece of his rhetoric
(as in the Gettysburg Address of 1863) and his policies. Since then, it has become a well-known statement
on human rights, particularly its second sentence:
We hold these truths to be self-evident, that all men are created equal, that they are endowed by their
Creator with certain unalienable Rights, that among these are Life, Liberty and the pursuit of Happiness.
This has been called "one of the best-known sentences in the English language", containing "the most potent
and consequential words in American history". The passage came to represent a moral standard to which
the United States should strive. This view was notably promoted by Abraham Lincoln, who considered the
Declaration to be the foundation of his political philosophy and argued that it is a statement of principles
through which the United States Constitution should be interpreted.
The U.S. Declaration of Independence inspired many other similar documents in other countries, the first
being the 1789 Declaration of Flanders issued during the Brabant Revolution in the Austrian Netherlands
(modern-day Belgium). It also served as the primary model for numerous declarations of independence across
Europe and Latin America, as well as Africa (Liberia) and Oceania (New Zealand) during the first half of the
19th century.'''
# Split the string into a list of words.
word_list = string_words.split()
# Create a list of word frequencies using list comprehension.
word_freq = [word_list.count(n) for n in word_list]
# Print the original string, the list of words, and pairs of words along with their frequencies.
print("String:\n {} \n".format(string_words))
print("List:\n {} \n".format(str(word_list)))
print("Pairs (Words and Frequencies:\n {}".format(str(list(zip(word_list, word_freq)))))
Sample Output:
String: United States Declaration of Independence From Wikipedia, the free encyclopedia The United States Declaration of Independence is the statement adopted by the Second Continental Congress meeting at the Pennsylvania State House (Independence Hall) in Philadelphia on July 4, 1776, which announced that the thirteen American colonies, then at war with the Kingdom of Great Britain, regarded themselves as thirteen independent sovereign states, no longer under British rule. These states would found a new nation – the United States of America. John Adams was a leader in pushing for independence, which was passed on July 2 with no opposing vote cast. A committee of five had already drafted the formal declaration, to be ready when Congress voted on independence. John Adams persuaded the committee to select Thomas Jefferson to compose the original draft of the document, which Congress would edit to produce the final version. The Declaration was ultimately a formal explanation of why Congress had voted on July 2 to declare independence from Great Britain, more than a year after the outbreak of the American Revolutionary War. The next day, Adams wrote to his wife Abigail: "The Second Day of July 1776, will be the most memorable Epocha, in the History of America." But Independence Day is actually celebrated on July 4, the date that the Declaration of Independence was approved. After ratifying the text on July 4, Congress issued the Declaration of Independence in several forms. It was initially published as the printed Dunlap broadside that was widely distributed and read to the public. The source copy used for this printing has been lost, and may have been a copy in Thomas Jefferson's hand.[5] Jefferson's original draft, complete with changes made by John Adams and Benjamin Franklin, and Jefferson's notes of changes made by Congress, are preserved at the Library of Congress. The best-known version of the Declaration is a signed copy that is displayed at the National Archives in Washington, D.C., and which is popularly regarded as the official document. This engrossed copy was ordered by Congress on July 19 and signed primarily on August 2. The sources and interpretation of the Declaration have been the subject of much scholarly inquiry. The Declaration justified the independence of the United States by listing colonial grievances against King George III, and by asserting certain natural and legal rights, including a right of revolution. Having served its original purpose in announcing independence, references to the text of the Declaration were few in the following years. Abraham Lincoln made it the centerpiece of his rhetoric (as in the Gettysburg Address of 1863) and his policies. Since then, it has become a well-known statement on human rights, particularly its second sentence: We hold these truths to be self-evident, that all men are created equal, that they are endowed by their Creator with certain unalienable Rights, that among these are Life, Liberty and the pursuit of Happiness. This has been called "one of the best-known sentences in the English language", containing "the most potent and consequential words in American history". The passage came to represent a moral standard to which the United States should strive. This view was notably promoted by Abraham Lincoln, who considered the Declaration to be the foundation of his political philosophy and argued that it is a statement of principles through which the United States Constitution should be interpreted. The U.S. Declaration of Independence inspired many other similar documents in other countries, the first being the 1789 Declaration of Flanders issued during the Brabant Revolution in the Austrian Netherlands (modern-day Belgium). It also served as the primary model for numerous declarations of independence across Europe and Latin America, as well as Africa (Liberia) and Oceania (New Zealand) during the first half of the 19th century. List: ['United', 'States', 'Declaration', 'of', 'Independence', 'From', 'Wikipedia,', 'the', 'free', 'encyclopedia', 'The', 'United', 'States', 'Declaration', 'of', 'Independence', 'is', 'the', 'statement', 'adopted', 'by', 'the', 'Second', 'Continental', 'Congress', 'meeting', 'at', 'the', 'Pennsylvania', 'State', 'House', '(Independence', 'Hall)', 'in', 'Philadelphia', 'on', 'July', '4,', '1776,', 'which', 'announced', 'that', 'the', 'thirteen', 'American', 'colonies,', 'then', 'at', 'war', 'with', 'the', 'Kingdom', 'of', 'Great', 'Britain,', 'regarded', 'themselves', 'as', 'thirteen', 'independent', 'sovereign', 'states,', 'no', 'longer', 'under', 'British', 'rule.', 'These', 'states', 'would', 'found', 'a', 'new', 'nation', '–', 'the', 'United', 'States', 'of', 'America.', 'John', 'Adams', 'was', 'a', 'leader', 'in', 'pushing', 'for', 'independence,', 'which', 'was', 'passed', 'on', 'July', '2', 'with', 'no', 'opposing', 'vote', 'cast.', 'A', 'committee', 'of', 'five', 'had', 'already', 'drafted', 'the', 'formal', 'declaration,', 'to', 'be', 'ready', 'when', 'Congress', 'voted', 'on', 'independence.', 'John', 'Adams', 'persuaded', 'the', 'committee', 'to', 'select', 'Thomas', 'Jefferson', 'to', 'compose', 'the', 'original', 'draft', 'of', 'the', 'document,', 'which', 'Congress', 'would', 'edit', 'to', 'produce', 'the', 'final', 'version.', 'The', 'Declaration', 'was', 'ultimately', 'a', 'formal', 'explanation', 'of', 'why', 'Congress', 'had', 'voted', 'on', 'July', '2', 'to', 'declare', 'independence', 'from', 'Great', 'Britain,', 'more', 'than', 'a', 'year', 'after', 'the', 'outbreak', 'of', 'the', 'American', 'Revolutionary', 'War.', 'The', 'next', 'day,', 'Adams', 'wrote', 'to', 'his', 'wife', 'Abigail:', '"The', 'Second', 'Day', 'of', 'July', '1776,', 'will', 'be', 'the', 'most', 'memorable', 'Epocha,', 'in', 'the', 'History', 'of', 'America."', 'But', 'Independence', 'Day', 'is', 'actually', 'celebrated', 'on', 'July', '4,', 'the', 'date', 'that', 'the', 'Declaration', 'of', 'Independence', 'was', 'approved.', 'After', 'ratifying', 'the', 'text', 'on', 'July', '4,', 'Congress', 'issued', 'the', 'Declaration', 'of', 'Independence', 'in', 'several', 'forms.', 'It', 'was', 'initially', 'published', 'as', 'the', 'printed', 'Dunlap', 'broadside', 'that', 'was', 'widely', 'distributed', 'and', 'read', 'to', 'the', 'public.', 'The', 'source', 'copy', 'used', 'for', 'this', 'printing', 'has', 'been', 'lost,', 'and', 'may', 'have', 'been', 'a', 'copy', 'in', 'Thomas', "Jefferson's", 'hand.[5]', "Jefferson's", 'original', 'draft,', 'complete', 'with', 'changes', 'made', 'by', 'John', 'Adams', 'and', 'Benjamin', 'Franklin,', 'and', "Jefferson's", 'notes', 'of', 'changes', 'made', 'by', 'Congress,', 'are', 'preserved', 'at', 'the', 'Library', 'of', 'Congress.', 'The', 'best-known', 'version', 'of', 'the', 'Declaration', 'is', 'a', 'signed', 'copy', 'that', 'is', 'displayed', 'at', 'the', 'National', 'Archives', 'in', 'Washington,', 'D.C.,', 'and', 'which', 'is', 'popularly', 'regarded', 'as', 'the', 'official', 'document.', 'This', 'engrossed', 'copy', 'was', 'ordered', 'by', 'Congress', 'on', 'July', '19', 'and', 'signed', 'primarily', 'on', 'August', '2.', 'The', 'sources', 'and', 'interpretation', 'of', 'the', 'Declaration', 'have', 'been', 'the', 'subject', 'of', 'much', 'scholarly', 'inquiry.', 'The', 'Declaration', 'justified', 'the', 'independence', 'of', 'the', 'United', 'States', 'by', 'listing', 'colonial', 'grievances', 'against', 'King', 'George', 'III,', 'and', 'by', 'asserting', 'certain', 'natural', 'and', 'legal', 'rights,', 'including', 'a', 'right', 'of', 'revolution.', 'Having', 'served', 'its', 'original', 'purpose', 'in', 'announcing', 'independence,', 'references', 'to', 'the', 'text', 'of', 'the', 'Declaration', 'were', 'few', 'in', 'the', 'following', 'years.', 'Abraham', 'Lincoln', 'made', 'it', 'the', 'centerpiece', 'of', 'his', 'rhetoric', '(as', 'in', 'the', 'Gettysburg', 'Address', 'of', '1863)', 'and', 'his', 'policies.', 'Since', 'then,', 'it', 'has', 'become', 'a', 'well-known', 'statement', 'on', 'human', 'rights,', 'particularly', 'its', 'second', 'sentence:', 'We', 'hold', 'these', 'truths', 'to', 'be', 'self-evident,', 'that', 'all', 'men', 'are', 'created', 'equal,', 'that', 'they', 'are', 'endowed', 'by', 'their', 'Creator', 'with', 'certain', 'unalienable', 'Rights,', 'that', 'among', 'these', 'are', 'Life,', 'Liberty', 'and', 'the', 'pursuit', 'of', 'Happiness.', 'This', 'has', 'been', 'called', '"one', 'of', 'the', 'best-known', 'sentences', 'in', 'the', 'English', 'language",', 'containing', '"the', 'most', 'potent', 'and', 'consequential', 'words', 'in', 'American', 'history".', 'The', 'passage', 'came', 'to', 'represent', 'a', 'moral', 'standard', 'to', 'which', 'the', 'United', 'States', 'should', 'strive.', 'This', 'view', 'was', 'notably', 'promoted', 'by', 'Abraham', 'Lincoln,', 'who', 'considered', 'the', 'Declaration', 'to', 'be', 'the', 'foundation', 'of', 'his', 'political', 'philosophy', 'and', 'argued', 'that', 'it', 'is', 'a', 'statement', 'of', 'principles', 'through', 'which', 'the', 'United', 'States', 'Constitution', 'should', 'be', 'interpreted.', 'The', 'U.S.', 'Declaration', 'of', 'Independence', 'inspired', 'many', 'other', 'similar', 'documents', 'in', 'other', 'countries,', 'the', 'first', 'being', 'the', '1789', 'Declaration', 'of', 'Flanders', 'issued', 'during', 'the', 'Brabant', 'Revolution', 'in', 'the', 'Austrian', 'Netherlands', '(modern-day', 'Belgium).', 'It', 'also', 'served', 'as', 'the', 'primary', 'model', 'for', 'numerous', 'declarations', 'of', 'independence', 'across', 'Europe', 'and', 'Latin', 'America,', 'as', 'well', 'as', 'Africa', '(Liberia)', 'and', 'Oceania', '(New', 'Zealand)', 'during', 'the', 'first', 'half', 'of', 'the', '19th', 'century.'] Pairs (Words and Frequencies: [('United', 6), ('States', 6), ('Declaration', 12), ('of', 30), ('Independence', 6), ('From', 1), ('Wikipedia,', 1), ('the', 49), ('free', 1), ('encyclopedia', 1), ('The', 9), ('United', 6), ('States', 6), ('Declaration', 12), ('of', 30), ('Independence', 6), ('is', 6), ('the', 49), ('statement', 3), ('adopted', 1), ('by', 8), ('the', 49), ('Second', 2), ('Continental', 1), ('Congress', 6), ('meeting', 1), ('at', 4), ('the', 49), ('Pennsylvania', 1), ('State', 1), ('House', 1), ('(Independence', 1), ('Hall)', 1), ('in', 13), ('Philadelphia', 1), ('on', 9), ('July', 7), ('4,', 3), ('1776,', 2), ('which', 6), ('announced', 1), ('that', 8), ('the', 49), ('thirteen', 2), ('American', 3), ('colonies,', 1), ('then', 1), ('at', 4), ('war', 1), ('with', 4), ('the', 49), ('Kingdom', 1), ('of', 30), ('Great', 2), ('Britain,', 2), ('regarded', 2), ('themselves', 1), ('as', 6), ('thirteen', 2), ('independent', 1), ('sovereign', 1), ('states,', 1), ('no', 2), ('longer', 1), ('under', 1), ('British', 1), ('rule.', 1), ('These', 1), ('states', 1), ('would', 2), ('found', 1), ('a', 10), ('new', 1), ('nation', 1), ('–', 1), ('the', 49), ('United', 6), ('States', 6), ('of', 30), ('America.', 1), ('John', 3), ('Adams', 4), ('was', 8), ('a', 10), ('leader', 1), ('in', 13), ('pushing', 1), ('for', 3), ('independence,', 2), ('which', 6), ('was', 8), ('passed', 1), ('on', 9), ('July', 7), ('2', 2), ('with', 4), ('no', 2), ('opposing', 1), ('vote', 1), ('cast.', 1), ('A', 1), ('committee', 2), ('of', 30), ('five', 1), ('had', 2), ('already', 1), ('drafted', 1), ('the', 49), ('formal', 2), ('declaration,', 1), ('to', 12), ('be', 5), ('ready', 1), ('when', 1), ('Congress', 6), ('voted', 2), ('on', 9), ('independence.', 1), ('John', 3), ('Adams', 4), ('persuaded', 1), ('the', 49), ('committee', 2), ('to', 12), ('select', 1), ('Thomas', 2), ('Jefferson', 1), ('to', 12), ('compose', 1), ('the', 49), ('original', 3), ('draft', 1), ('of', 30), ('the', 49), ('document,', 1), ('which', 6), ('Congress', 6), ('would', 2), ('edit', 1), ('to', 12), ('produce', 1), ('the', 49), ('final', 1), ('version.', 1), ('The', 9), ('Declaration', 12), ('was', 8), ('ultimately', 1), ('a', 10), ('formal', 2), ('explanation', 1), ('of', 30), ('why', 1), ('Congress', 6), ('had', 2), ('voted', 2), ('on', 9), ('July', 7), ('2', 2), ('to', 12), ('declare', 1), ('independence', 3), ('from', 1), ('Great', 2), ('Britain,', 2), ('more', 1), ('than', 1), ('a', 10), ('year', 1), ('after', 1), ('the', 49), ('outbreak', 1), ('of', 30), ('the', 49), ('American', 3), ('Revolutionary', 1), ('War.', 1), ('The', 9), ('next', 1), ('day,', 1), ('Adams', 4), ('wrote', 1), ('to', 12), ('his', 4), ('wife', 1), ('Abigail:', 1), ('"The', 1), ('Second', 2), ('Day', 2), ('of', 30), ('July', 7), ('1776,', 2), ('will', 1), ('be', 5), ('the', 49), ('most', 2), ('memorable', 1), ('Epocha,', 1), ('in', 13), ('the', 49), ('History', 1), ('of', 30), ('America."', 1), ('But', 1), ('Independence', 6), ('Day', 2), ('is', 6), ('actually', 1), ('celebrated', 1), ('on', 9), ('July', 7), ('4,', 3), ('the', 49), ('date', 1), ('that', 8), ('the', 49), ('Declaration', 12), ('of', 30), ('Independence', 6), ('was', 8), ('approved.', 1), ('After', 1), ('ratifying', 1), ('the', 49), ('text', 2), ('on', 9), ('July', 7), ('4,', 3), ('Congress', 6), ('issued', 2), ('the', 49), ('Declaration', 12), ('of', 30), ('Independence', 6), ('in', 13), ('several', 1), ('forms.', 1), ('It', 2), ('was', 8), ('initially', 1), ('published', 1), ('as', 6), ('the', 49), ('printed', 1), ('Dunlap', 1), ('broadside', 1), ('that', 8), ('was', 8), ('widely', 1), ('distributed', 1), ('and', 15), ('read', 1), ('to', 12), ('the', 49), ('public.', 1), ('The', 9), ('source', 1), ('copy', 4), ('used', 1), ('for', 3), ('this', 1), ('printing', 1), ('has', 3), ('been', 4), ('lost,', 1), ('and', 15), ('may', 1), ('have', 2), ('been', 4), ('a', 10), ('copy', 4), ('in', 13), ('Thomas', 2), ("Jefferson's", 3), ('hand.[5]', 1), ("Jefferson's", 3), ('original', 3), ('draft,', 1), ('complete', 1), ('with', 4), ('changes', 2), ('made', 3), ('by', 8), ('John', 3), ('Adams', 4), ('and', 15), ('Benjamin', 1), ('Franklin,', 1), ('and', 15), ("Jefferson's", 3), ('notes', 1), ('of', 30), ('changes', 2), ('made', 3), ('by', 8), ('Congress,', 1), ('are', 4), ('preserved', 1), ('at', 4), ('the', 49), ('Library', 1), ('of', 30), ('Congress.', 1), ('The', 9), ('best-known', 2), ('version', 1), ('of', 30), ('the', 49), ('Declaration', 12), ('is', 6), ('a', 10), ('signed', 2), ('copy', 4), ('that', 8), ('is', 6), ('displayed', 1), ('at', 4), ('the', 49), ('National', 1), ('Archives', 1), ('in', 13), ('Washington,', 1), ('D.C.,', 1), ('and', 15), ('which', 6), ('is', 6), ('popularly', 1), ('regarded', 2), ('as', 6), ('the', 49), ('official', 1), ('document.', 1), ('This', 3), ('engrossed', 1), ('copy', 4), ('was', 8), ('ordered', 1), ('by', 8), ('Congress', 6), ('on', 9), ('July', 7), ('19', 1), ('and', 15), ('signed', 2), ('primarily', 1), ('on', 9), ('August', 1), ('2.', 1), ('The', 9), ('sources', 1), ('and', 15), ('interpretation', 1), ('of', 30), ('the', 49), ('Declaration', 12), ('have', 2), ('been', 4), ('the', 49), ('subject', 1), ('of', 30), ('much', 1), ('scholarly', 1), ('inquiry.', 1), ('The', 9), ('Declaration', 12), ('justified', 1), ('the', 49), ('independence', 3), ('of', 30), ('the', 49), ('United', 6), ('States', 6), ('by', 8), ('listing', 1), ('colonial', 1), ('grievances', 1), ('against', 1), ('King', 1), ('George', 1), ('III,', 1), ('and', 15), ('by', 8), ('asserting', 1), ('certain', 2), ('natural', 1), ('and', 15), ('legal', 1), ('rights,', 2), ('including', 1), ('a', 10), ('right', 1), ('of', 30), ('revolution.', 1), ('Having', 1), ('served', 2), ('its', 2), ('original', 3), ('purpose', 1), ('in', 13), ('announcing', 1), ('independence,', 2), ('references', 1), ('to', 12), ('the', 49), ('text', 2), ('of', 30), ('the', 49), ('Declaration', 12), ('were', 1), ('few', 1), ('in', 13), ('the', 49), ('following', 1), ('years.', 1), ('Abraham', 2), ('Lincoln', 1), ('made', 3), ('it', 3), ('the', 49), ('centerpiece', 1), ('of', 30), ('his', 4), ('rhetoric', 1), ('(as', 1), ('in', 13), ('the', 49), ('Gettysburg', 1), ('Address', 1), ('of', 30), ('1863)', 1), ('and', 15), ('his', 4), ('policies.', 1), ('Since', 1), ('then,', 1), ('it', 3), ('has', 3), ('become', 1), ('a', 10), ('well-known', 1), ('statement', 3), ('on', 9), ('human', 1), ('rights,', 2), ('particularly', 1), ('its', 2), ('second', 1), ('sentence:', 1), ('We', 1), ('hold', 1), ('these', 2), ('truths', 1), ('to', 12), ('be', 5), ('self-evident,', 1), ('that', 8), ('all', 1), ('men', 1), ('are', 4), ('created', 1), ('equal,', 1), ('that', 8), ('they', 1), ('are', 4), ('endowed', 1), ('by', 8), ('their', 1), ('Creator', 1), ('with', 4), ('certain', 2), ('unalienable', 1), ('Rights,', 1), ('that', 8), ('among', 1), ('these', 2), ('are', 4), ('Life,', 1), ('Liberty', 1), ('and', 15), ('the', 49), ('pursuit', 1), ('of', 30), ('Happiness.', 1), ('This', 3), ('has', 3), ('been', 4), ('called', 1), ('"one', 1), ('of', 30), ('the', 49), ('best-known', 2), ('sentences', 1), ('in', 13), ('the', 49), ('English', 1), ('language",', 1), ('containing', 1), ('"the', 1), ('most', 2), ('potent', 1), ('and', 15), ('consequential', 1), ('words', 1), ('in', 13), ('American', 3), ('history".', 1), ('The', 9), ('passage', 1), ('came', 1), ('to', 12), ('represent', 1), ('a', 10), ('moral', 1), ('standard', 1), ('to', 12), ('which', 6), ('the', 49), ('United', 6), ('States', 6), ('should', 2), ('strive.', 1), ('This', 3), ('view', 1), ('was', 8), ('notably', 1), ('promoted', 1), ('by', 8), ('Abraham', 2), ('Lincoln,', 1), ('who', 1), ('considered', 1), ('the', 49), ('Declaration', 12), ('to', 12), ('be', 5), ('the', 49), ('foundation', 1), ('of', 30), ('his', 4), ('political', 1), ('philosophy', 1), ('and', 15), ('argued', 1), ('that', 8), ('it', 3), ('is', 6), ('a', 10), ('statement', 3), ('of', 30), ('principles', 1), ('through', 1), ('which', 6), ('the', 49), ('United', 6), ('States', 6), ('Constitution', 1), ('should', 2), ('be', 5), ('interpreted.', 1), ('The', 9), ('U.S.', 1), ('Declaration', 12), ('of', 30), ('Independence', 6), ('inspired', 1), ('many', 1), ('other', 2), ('similar', 1), ('documents', 1), ('in', 13), ('other', 2), ('countries,', 1), ('the', 49), ('first', 2), ('being', 1), ('the', 49), ('1789', 1), ('Declaration', 12), ('of', 30), ('Flanders', 1), ('issued', 2), ('during', 2), ('the', 49), ('Brabant', 1), ('Revolution', 1), ('in', 13), ('the', 49), ('Austrian', 1), ('Netherlands', 1), ('(modern-day', 1), ('Belgium).', 1), ('It', 2), ('also', 1), ('served', 2), ('as', 6), ('the', 49), ('primary', 1), ('model', 1), ('for', 3), ('numerous', 1), ('declarations', 1), ('of', 30), ('independence', 3), ('across', 1), ('Europe', 1), ('and', 15), ('Latin', 1), ('America,', 1), ('as', 6), ('well', 1), ('as', 6), ('Africa', 1), ('(Liberia)', 1), ('and', 15), ('Oceania', 1), ('(New', 1), ('Zealand)', 1), ('during', 2), ('the', 49), ('first', 2), ('half', 1), ('of', 30), ('the', 49), ('19th', 1), ('century.', 1)]
Explanation:
Here's a brief explanation of the above code:
- string_words: A long string containing a passage about the United States Declaration of Independence.
- word_list: The string is split into a list of words.
- word_freq: A list comprehension that creates a list of word frequencies by counting the occurrences of each word in 'word_list'.
- The code then prints the original string, the list of words, and a list of word-frequency pairs.
Visual Presentation:
Flowchart:
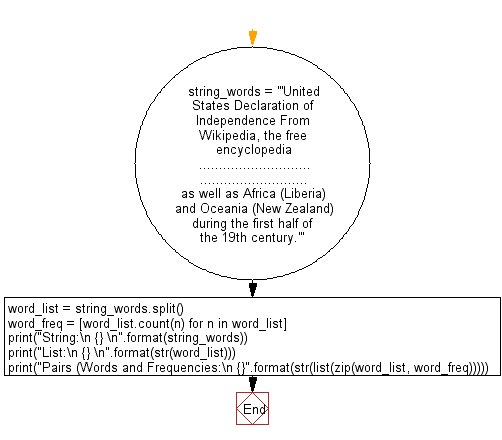
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to create the combinations of 3 digit combo.
Next: Write a Python program to count the number of each character of a text file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics