Python: Read the mass data and find the number of islands
Python Basic - 1: Exercise-57 with Solution
Island Counter in Grid
There are 10 vertical and horizontal squares on a plane. Each square is painted blue and green. Blue represents the sea, and green represents the land. When two green squares are in contact with the top and bottom, or right and left, they are said to be ground. The area created by only one green square is called "island". For example, there are five islands in the figure below.
Write a Python program to read the mass data and find the number of islands.
Input:
A single data set is represented by 10 rows of 10 numbers representing green squares as 1 and blue squares as zeros.
1100000111
1000000111
0000000111
0010001000
0000011100
0000111110
0001111111
1000111110
1100011100
1110001000
Number of islands:
5
Visual Presentation:
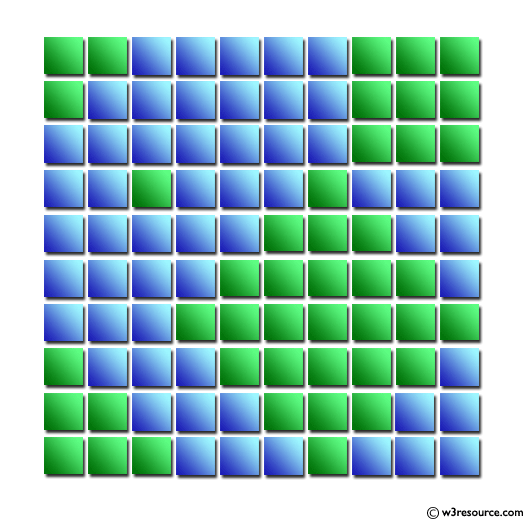
Sample Solution:
Python Code:
# Initialize counter variable 'c' to 0
c = 0
# Define a recursive function 'f' with parameters x, y, and z
def f(x, y, z):
# Check conditions for valid coordinates and if the square is part of the island ('1')
if 0 <= y < 10 and 0 <= z < 10 and x[z][y] == '1':
# Change the square to '0' to mark it as visited
x[z][y] = '0'
# Recursively call 'f' for neighboring squares
for dy, dz in [[-1, 0], [1, 0], [0, -1], [0, 1]]:
f(x, y + dy, z + dz)
# Print a statement instructing the user to input 10 rows of 10 numbers representing the island and sea
print("Input 10 rows of 10 numbers representing green squares (island) as 1 and blue squares (sea) as zeros")
# Run an infinite loop
while 1:
try:
# If 'c' is not 0, prompt the user to input (used for multiple test cases)
if c:
input()
except:
# Break the loop if an exception occurs (typically EOF)
break
# Read 10 rows of 10 characters each to represent the island and sea
x = [list(input()) for _ in [0] * 10]
# Set 'c' to 1 to indicate subsequent iterations
c = 1
# Initialize counter 'b' to 0 to count the number of islands
b = 0
# Nested loops to iterate through each square in the grid
for i in range(10):
for j in range(10):
# Check if the square is part of an island ('1')
if x[j][i] == '1':
# Increment the island counter 'b'
b += 1
# Call the recursive function 'f' to mark the connected island squares
f(x, i, j)
# Print the number of islands in the input grid
print("Number of islands:")
print(b)
Sample Output:
Input 10 rows of 10 numbers representing green squares (island) as 1 and blue squares (sea) as zeros 1100000111 1000000111 0000000111 0010001000 0000011100 0000111110 0001111111 1000111110 1100011100 1110001000 Number of islands: 5
Explanation:
Here is a breakdown of the above Python code:
- First initialize a counter variable 'c' to 0.
- Define a recursive function 'f' to traverse and mark connected island squares.
- Print a statement instructing the user to input a grid of 10 rows by 10 numbers.
- Enter an infinite loop with a try-except block to handle user input.
- Read 10 rows of 10 characters each to represent the island ('1') and sea ('0').
- Set 'c' to 1 for subsequent iterations.
- Initialize a counter 'b' to 0 to count the number of islands.
- Use nested loops to iterate through each square in the grid.
- If a square is part of an island ('1'), increment the island counter 'b' and call the recursive function 'f' to mark connected island squares.
- Print the total number of islands in the input grid.
Flowchart:
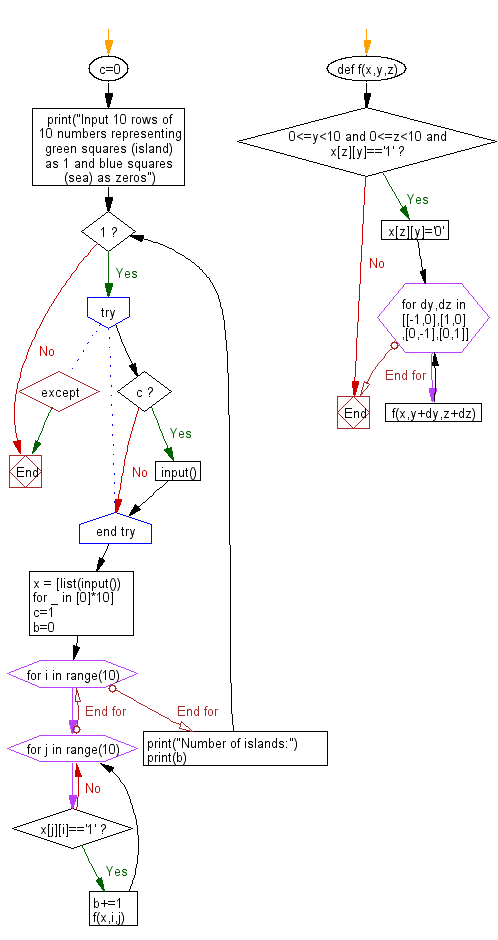
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to sum of all numerical values (positive integers) embedded in a sentence.
Next: Write a Python program to restore the original string by entering the compressed string with this rule. However, the # character does not appear in the restored character string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/basic/python-basic-1-exercise-57.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics