Python: Sum of all numerical values embedded in a sentence
Sum of Numbers in Text
Write a Python program to sum all numerical values (positive integers) embedded in a sentence.
Input:
Sentences with positive integers are given over multiple lines. Each line is a character string containing one-byte alphanumeric characters, symbols, spaces, or an empty line. However the input is 80 characters or less per line and the sum is 10,000 or less.
Sample Solution:
Python Code:
# Import sys and re modules
import sys
import re
# Define a function named 'test' that takes a string 'stri' as a parameter
def test(stri):
# Print statement to instruct the user to input text and numeric values (terminate with )
print("Input some text and numeric values ( to exit):")
# Use regex to find all numeric values in the input string and sum them
numeric_sum = sum(map(int, re.findall(r"[0-9]{1,5}", stri)))
# Print the sum of the numeric values
print("Sum of the numeric values: ", numeric_sum)
# Test the 'test' function with different input strings
print(test("sd1fdsfs23 dssd56"))
print(test("15apple2banana"))
print(test("flowers5fruit5"))
Sample Output:
Input some text and numeric values (to exit): Sum of the numeric values: 80 None Input some text and numeric values ( to exit): Sum of the numeric values: 17 None Input some text and numeric values ( to exit): Sum of the numeric values: 10 None
Explanation:
Here is a breakdown of the above Python code:
- Import the "sys" and "re" modules.
- Define a function named "test()" that takes a string parameter 'stri'.
- Print a statement instructing the user to input text and numeric values, indicating termination with <ctrl-d>.
- Use the re.findall function with a regex pattern to find all numeric values (1 to 5 digits) in the input string.
- Map the found numeric values to integers and calculate their sum using "sum()".
- Print the sum of the numeric values.
- Test the "test()" function with three different input strings. Note: The function should not return anything (hence, print(test(...)) may print None).
Visual Presentation:
Flowchart:
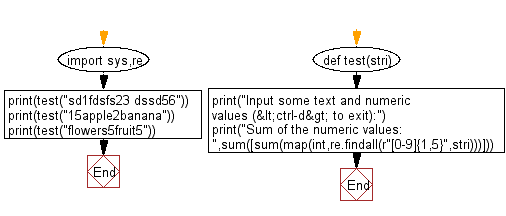
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to test AB and CD are orthogonal or not.
Next: Write a Python program to read the mass data and find the number of islands.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics