Python: Reads n digits chosen from 0 to 9 and prints the number of combinations where the sum of the digits equals to another given number(s)
Sum of Digit Combinations
Write a Python program that reads n digits (given) chosen from 0 to 9 and prints the number of combinations where the sum of the digits equals another given number (s). Do not use the same digits in a combination.
Input:
Two integers as number of combinations and their sum by a single space in a line. Input 0 0 to exit.
Sample Solution:
Python Code:
# Import the 'itertools' module
import itertools
# Prompt the user to input the number of combinations and sum, input 0 0 to exit
print("Input number of combinations and sum, input 0 0 to exit:")
# Infinite loop for continuous input until the user enters 0 0
while True:
# Take user input for the number of combinations and sum, and convert to integers
x, y = map(int, input().split())
# Check if the user wants to exit the loop
if x == 0 and y == 0:
break
# Generate all combinations of 'x' elements from the range 0 to 9
s = list(itertools.combinations(range(10), x))
# Initialize a counter variable to count the valid combinations
ctr = 0
# Iterate through each combination
for i in s:
# Check if the sum of the current combination is equal to the target sum 'y'
if sum(i) == y:
ctr += 1
# Print the total count of valid combinations
print(ctr)
Sample Output:
Input number of combinations and sum, input 0 0 to exit: 5 6 2 4 0 0 2
Explanation:
Here is a breakdown of the above Python code:
- First the code imports the 'itertools' module.
- It prompts the user to input the number of combinations and sum, with the option to input 0 0 to exit.
- The code enters an infinite loop to continuously take input until the user enters 0 0.
- User input for the number of combinations and sum is taken and converted to integers.
- It generates all combinations of 'x' elements from the range 0 to 9.
- The code iterates through each combination, checking if the sum equals the target sum 'y'.
- The total count of valid combinations is printed after exiting the loop.
Flowchart:
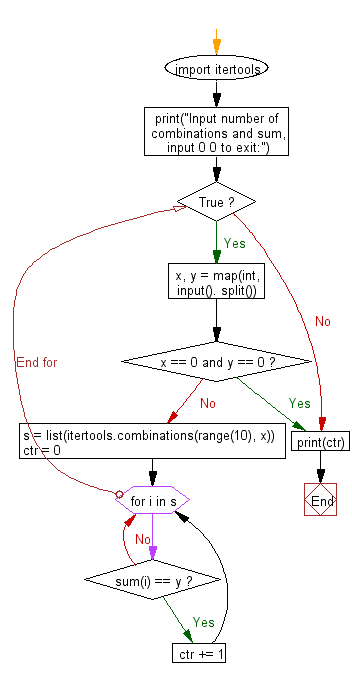
For more Practice: Solve these Related Problems:
- Write a Python program to count the combinations of n digits where the product equals a given number without reusing digits.
- Write a Python program to generate all unique permutations of n digits that sum to a given target.
- Write a Python program to partition a set of digits into two groups that have equal sums.
- Write a Python program to determine the maximum sum achievable by combining a set of given digits in any order.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program which reads a text (only alphabetical characters and spaces.) and prints two words. The first one is the word which is arise most frequently in the text. The second one is the word which has the maximum number of letters.
Next: Write a Python program which reads the two adjoined sides and the diagonal of a parallelogram and check whether the parallelogram is a rectangle or a rhombus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.