Python: Compute and print sum of two given integers
Python Basic - 1: Exercise-41 with Solution
Write a Python program to compute and print the sum of two given integers (greater or equal to zero). In the event that the given integers or the sum exceed 80 digits, print "overflow".
Sample Solution:
Python Code:
# Print statement to prompt the user to input the first integer
print("Input first integer:")
# Take user input and convert it to an integer
x = int(input())
# Print statement to prompt the user to input the second integer
print("Input second integer:")
# Take user input and convert it to an integer
y = int(input())
# Check if any of the integers or their sum exceeds 10^80
if x >= 10 ** 80 or y >= 10 ** 80 or x + y >= 10 ** 80:
print("Overflow!") # Print overflow message if the condition is met
else:
print("Sum of the two integers: ", x + y) # Print the sum if no overflow occurs
Sample Output:
Input first integer: 25 Input second integer: 22 Sum of the two integers: 47
Explanation:
The above Python program prompts the user to input two integers, 'x' and 'y'. It then checks whether any of these integers or their sum exceeds the value of 10^80. If the condition is met, indicating that the result is too large to be represented, it prints "Overflow!". Otherwise, it calculates and prints the sum of the two integers. The purpose is to prevent integer overflow for extremely large values.
Flowchart:
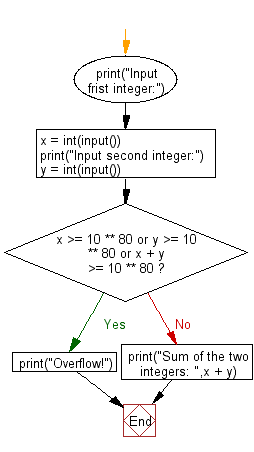
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to check if a point (x,y) is in a triangle or not. There is a triangle formed by three points.
Next: Write a Python program that accepts six numbers as input and sorts them in descending order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/basic/python-basic-1-exercise-41.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics