Python: Find the number of combinations of a,b,c and d
Sum Combinations Counter
Write a Python program that reads an integer n and finds the number of combinations of a,b,c and d (0 = a,b,c,d = 9) where (a + b + c + d) will be equal to n.
Input:
n (1 ≤ n ≤ 50)
Input the number(n): 15
Number of combinations: 592
Sample Solution:
Python Code:
# Import the itertools module to work with iterators and combinatorial functions
import itertools
# Prompt the user to input a number 'n'
print("Input the number(n):")
# Read the user input and convert it to an integer
n = int(input())
# Initialize the variable 'result' to store the count of combinations
result = 0
# Iterate over all combinations of (i, j, k) where i, j, k are in the range [0, 9]
for (i, j, k) in itertools.product(range(10), range(10), range(10)):
# Check if the sum of i, j, and k lies between 0 and 9 (inclusive)
result += (0 <= n - (i + j + k) <= 9)
# Print the number of combinations that satisfy the condition
print("Number of combinations:", result)
Sample Output:
Input the number(n): 15 Number of combinations: 592
Explanation:
The above Python code prompts the user to input a number 'n' and then counts the number of combinations of three digits (i, j, k), where each digit ranges from 0 to 9, such that their sum is equal to 'n'. The code utilizes the itertools module to generate all possible combinations of (i, j, k) and increments a counter 'result' whenever the sum of these digits falls between 0 and 9 (inclusive).
Finally, it prints the count of combinations that satisfy the given condition.
Flowchart:
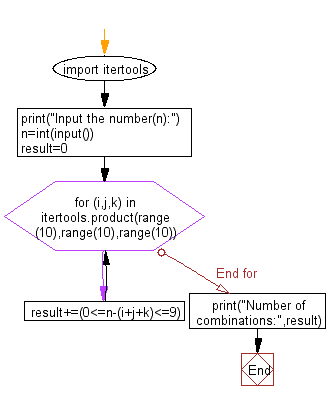
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to compute the amount of the debt in n months. The borrowing amount is $100,000 and the loan adds 5% interest of the debt and rounds it to the nearest 1,000 above month by month.
Next: Write a Python program to print the number of prime numbers which are less than or equal to an given integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics