Python: Find heights of the top three building in descending order from eight given buildings
Top Three Building Heights
Write a Python program to find the heights of the top three buildings in descending order from eight given buildings.
Input:
0 ≤ height of building (integer) ≤ 10,000
Visual Presentation:
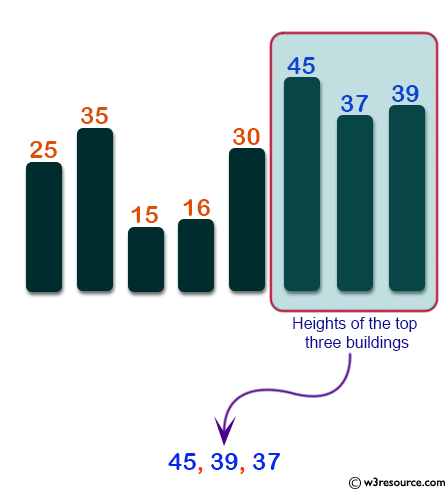
Sample Solution:
Python Code:
# Print statement to prompt the user to input the heights of eight buildings
print("Input the heights of eight buildings:")
# List comprehension to take input for the heights of eight buildings
l = [int(input()) for i in range(8)]
# Print statement to display the heights of the top three buildings
print("Heights of the top three buildings:")
# Sorting the list of building heights in ascending order
l = sorted(l)
# Printing the top three building heights in descending order using slicing and the 'sep' parameter for newlines
print(*l[:4:-1], sep='\n')
Sample Output:
Input the heights of eight buildings: 25 35 15 16 30 45 37 39 Heights of the top three buildings: 45 39 37
Explanation:
Here is the breakdown of the above Python exercise:
- The program starts by printing a statement prompting the user to input the heights of eight buildings.
- It uses list comprehension to create a list (l) containing the heights of eight buildings. The heights are converted to integers using int(input()).
- Another print statement is used to indicate that the program will display the heights of the top three buildings.
- The list of building heights (l) is sorted in ascending order.
- The heights of the top three buildings are printed in descending order using slicing (l[:4:-1]). The sep='\n' parameter ensures that each height is printed on a new line.
Flowchart:
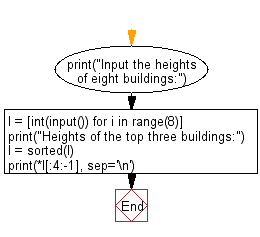
For more Practice: Solve these Related Problems:
- Write a Python program to find the top K highest buildings from a list of building heights.
- Write a Python program to compute the median building height from a provided list of heights.
- Write a Python program to determine the largest gap between consecutive building heights after sorting.
- Write a Python program to identify the second tallest building from a given list of building heights.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to count the number of carry operations for each of a set of addition problems.
Next: Write a Python program to compute the digit number of sum of two given integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics