Python: Compute the summation of the absolute difference of all distinct pairs in a given array
Absolute Pairwise Difference
Write a Python program to compute the summation of the absolute difference of all distinct pairs in a given array (non-decreasing order).
Sample array: [1, 2, 3]
Then all the distinct pairs will be:
1 2
1 3
2 3
Sample Solution:
Python Code:
# Define a function 'sum_distinct_pairs' that calculates the sum of distinct pairs.
def sum_distinct_pairs(arr):
# Initialize the result variable to store the sum.
result = 0
# Initialize the index variable.
i = 0
# Iterate through the array elements.
while i < len(arr):
# Calculate the contribution of the current element to the sum.
result += i * arr[i] - (len(arr) - i - 1) * arr[i]
# Increment the index.
i += 1
# Return the final result.
return result
# Test the 'sum_distinct_pairs' function with different arrays and print the results.
print(sum_distinct_pairs([1, 2, 3]))
print(sum_distinct_pairs([1, 4, 5]))
Sample Output:
4 8
Explanation:
The above Python code defines a function named "sum_distinct_pairs()" that calculates the sum of distinct pairs for a given list of numbers. Here's a brief explanation:
- Function Definition:
- def sum_distinct_pairs(arr):: Define a function named sum_distinct_pairs that takes a list of numbers 'arr' as input.
- Initialize Variables:
- result = 0: Initialize a variable 'result' to store the sum of distinct pairs.
- i = 0: Initialize an index variable to iterate through the array.
- Iterate Through Array:
- while i < len(arr):: Start a while loop to iterate through the elements of the array.
- Calculate Contribution to Sum:
- result += i arr[i] - (len(arr) - i - 1) arr[i]: Calculate the contribution of the current element to the sum based on the given formula.
- Increment Index:
- i += 1: Increment the index to move to the next element in the array.
- Return Result:
- return result: Return the final sum of distinct pairs.
- Function Testing:
- print(sum_distinct_pairs([1, 2, 3])): Test the "sum_distinct_pairs()" function with a specific array and print the result.
- print(sum_distinct_pairs([1, 4, 5])): Test the function with another array and print the result.
Visual Presentation:
Flowchart:
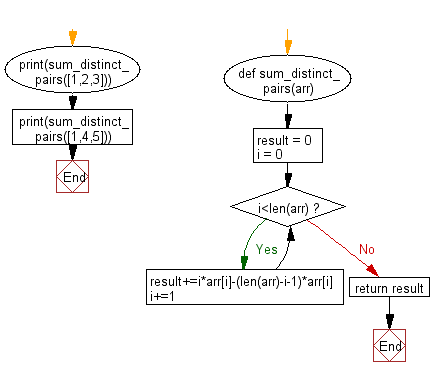
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find the digits which are absent in a given mobile number.
Next: Write a Python program to find the type of the progression (arithmetic progression/geometric progression) and the next successive member of a given three successive members of a sequence.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics