Python: Find the number of notes against a specified amount
Notes Count from Amount
Write a Python program to find the number of notes (Samples of notes: 10, 20, 50, 100, 200, 500) against an amount.
Range - Number of notes(n) : n (1 ≤ n ≤ 1000000).
Visual Presentation:
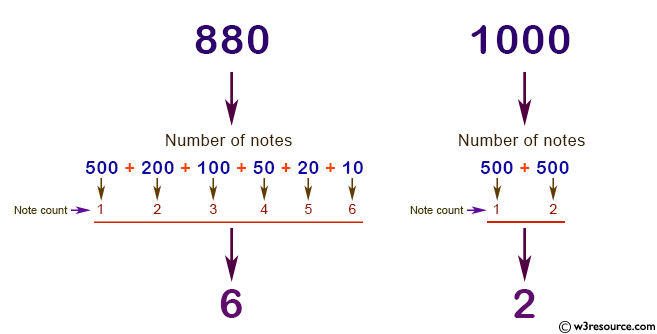
Sample Solution:
Python Code:
# Define a function 'no_notes' that calculates the minimum number of notes required
# to represent a given amount 'a' using denominations of 500, 200, 100, 50, 20, and 10.
def no_notes(a):
# List of denominations in descending order.
Q = [500, 200, 100, 50, 20, 10]
# Initialize a variable 'x' to 0.
x = 0
# Iterate over each denomination in the list.
for i in range(6):
q = Q[i] # Get the current denomination.
x += int(a / q) # Add the count of notes for the current denomination to 'x'.
a = int(a % q) # Update the remaining amount after using the current denomination.
# Check if there is any remaining amount not covered by the available denominations.
if a > 0:
x = -1 # If there is a remainder, set 'x' to -1.
return x # Return the minimum number of notes required.
# Test the 'no_notes' function with different amounts and print the results.
print(no_notes(880))
print(no_notes(1000))
Sample Output:
6 2
Explanation:
The above Python code defines a function "no_notes()" that calculates the minimum number of banknotes required to represent a given amount 'a' using denominations of 500, 200, 100, 50, 20, and 10. Here's a brief explanation:
- Function Definition:
- def no_notes(a):: Define a function named "no_notes" that takes an integer 'a' as input.
- Denomination List:
- Q = [500, 200, 100, 50, 20, 10]: Initialize a list 'Q' with denominations in descending order.
- Initialization:
- x = 0: Initialize a variable x to 0 to count the number of notes required.
- Iteration over Denominations:
- Iterate over each denomination in the list using a for loop.
- for i in range(6):: Loop through denominations from the largest to the smallest.
- q = Q[i]: Get the current denomination.
- x += int(a / q): Calculate the count of notes for the current denomination and add it to 'x'.
- a = int(a % q): Update the remaining amount after using the current denomination.
- Check Remaining Amount:
- if a > 0:: Check if there is any remaining amount not covered by the available denominations.
- x = -1: If there is a remainder, set 'x' to -1, indicating the amount cannot be represented with the available denominations.
- Return Result:
- return x: Return the minimum number of notes required to represent the amount 'a'.
- Function Testing:
- Test the no_notes function with different amounts (880 and 1000).
- Print the results of the function calls.
Flowchart:
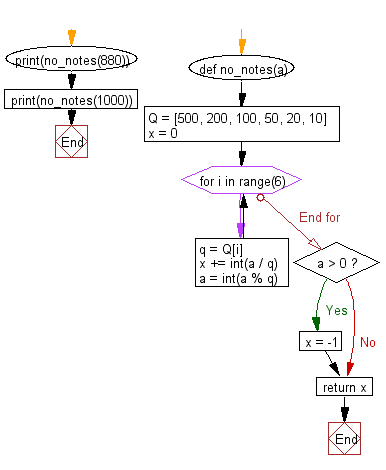
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find the number of zeros at the end of a factorial of a given positive number.
Next: Write a Python program to create a sequence where the first four members of the sequence are equal to one, and each successive term of the sequence is equal to the sum of the four previous ones. Find the Nth member of the sequence.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics