Python: Find the median among three given numbers
Find Median of Three
Write a Python program to find the median among three given numbers.
Visual Presentation:
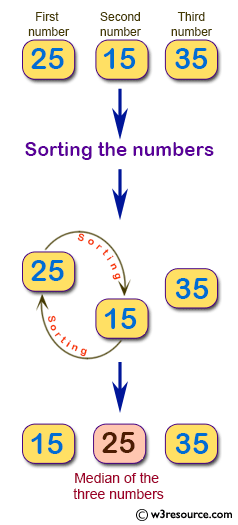
Sample Solution:
Python Code:
# Input the first number from the user
x = input("Input the first number: ")
# Input the second number from the user
y = input("Input the second number: ")
# Input the third number from the user
z = input("Input the third number: ")
# Print a message indicating that the program will calculate the median
print("Median of the above three numbers -")
# Check conditions to determine the median and print the result
if y < x and x < z:
print(x)
elif z < x and x < y:
print(x)
elif z < y and y < x:
print(y)
elif x < y and y < z:
print(y)
elif y < z and z < x:
print(z)
elif x < z and z < y:
print(z)
Sample Output:
Input the first number 25 Input the second number 15 Input the third number 35 Median of the above three numbers - 25
Explanation:
The above Python code takes three numbers as input from the user and calculates the median among them. Here's a brief explanation:
- Input the first number (x) from the user.
- Input the second number (y) from the user.
- Input the third number (z) from the user.
- Print a message indicating that the program will calculate the median of the three numbers.
- Check conditions to determine the median and print the result:
- If y is less than x and x is less than z, print x.
- If z is less than x and x is less than y, print x.
- If z is less than y and y is less than x, print y.
- If x is less than y and y is less than z, print y.
- If y is less than z and z is less than x, print z.
- If x is less than z and z is less than y, print z.
Flowchart:
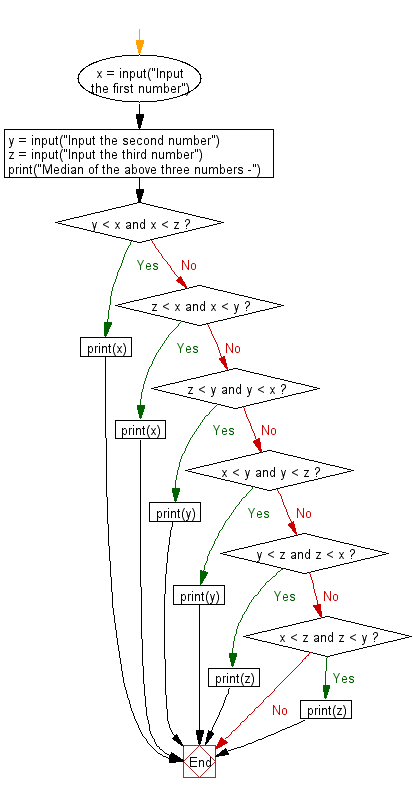
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to get all strobogrammatic numbers that are of length n.
Next: Write a Python program to find the value of n where n degrees of number 2 are written sequentially in a line without spaces.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics