Python Exercises: Check square root and cube root of a number
Cube and Square Root Match
A Python list contains two positive integers. Write a Python program to check whether the cube root of the first number is equal to the square root of the second number.
Sample Data:
([8, 4]) -> True
([64, 16]) -> True
([64, 36]) -> False
Sample Solution-1:
Python Code:
# Function to check if the square root of the first number is equal to the cube root of the second number.
def test(nums):
# Extract the two numbers from the list.
x = nums[0]
y = nums[1]
# Calculate the square root of y.
t = y**0.5
# Check if the square of the square root is equal to the cube of the first number.
if(x == t*t*t):
return True
else:
return False
# Example usage of the function with different lists of positive numbers.
nums = [8, 4]
print("Original list of positive numbers:")
print(nums)
print("Check square root and cube root of the said numbers:")
print(test(nums))
nums = [64, 16]
print("\nOriginal list of positive numbers:")
print(nums)
print("Check square root and cube root of the said numbers:")
print(test(nums))
nums = [64, 36]
print("\nOriginal list of positive numbers:")
print(nums)
print("Check square root and cube root of the said numbers:")
print(test(nums))
Sample Output:
Original list of positive numbers: [8, 4] True Check square root and cube root of the said numbers: Original list of positive numbers: [64, 16] Check square root and cube root of the said numbers: True Original list of positive numbers: [64, 36] Check square root and cube root of the said numbers: False
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- def test(nums):: Defines a function named "test()" that takes a list of two numbers (nums) as input.
- Variable assignment:
- x = nums[0]: Assigns the first element of the list to variable 'x'.
- y = nums[1]: Assigns the second element of the list to variable 'y'.
- Square root calculation:
- t = y**0.5: Calculates the square root of 'y' and assigns it to the variable 't'.
- Conditional check:
- if(x == t*t*t):: Checks if the cube of the square root (t) is equal to the cube of x.
- Return Statement:
- return True or return False: Returns 'True' if the condition is satisfied, otherwise 'False'.
Flowchart:
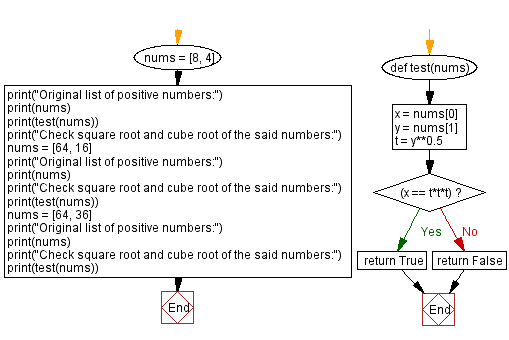
Sample Solution-2:
Python Code:
# Function to check if the cube root of the square root of the second number is equal to the first number.
def test(nums):
# Check if the cube root of the square root of the second number is equal to the first number.
return (((nums[1] ** 0.5) ** 3) == nums[0])
# Example usage of the function with different lists of positive numbers.
nums = [8, 4]
print("Original list of positive numbers:")
print(nums)
print("Check square root and cube root of the said numbers:")
print(test(nums))
nums = [64, 16]
print("\nOriginal list of positive numbers:")
print(nums)
print("Check square root and cube root of the said numbers:")
print(test(nums))
nums = [64, 36]
print("\nOriginal list of positive numbers:")
print(nums)
print("Check square root and cube root of the said numbers:")
print(test(nums))
Sample Output:
Original list of positive numbers: [8, 4] True Check square root and cube root of the said numbers: Original list of positive numbers: [64, 16] Check square root and cube root of the said numbers: True Original list of positive numbers: [64, 36] Check square root and cube root of the said numbers: False
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- def test(nums):: Defines a function named "test()" that takes a list of two numbers (nums) as input.
- Conditional check:
- return (((nums[1] 0.5) 3) == nums[0]): Checks if the cube root of the square root of the second number is equal to the first number.
- Example usage:
- Demonstrates the usage of the function with different lists of positive numbers and prints the results.
Flowchart:
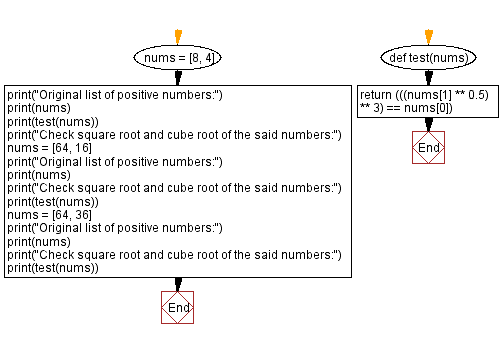
For more Practice: Solve these Related Problems:
- Write a Python program to verify if the cube root of the first number in a list equals the square root of the second number.
- Write a Python program to calculate the cube root of one number and the square root of another and compare the results.
- Write a Python program to check if a two-element list satisfies the condition where the cube root of the first equals the square root of the second.
- Write a Python program to determine whether the cube root of an integer matches the square root of another integer from an input list.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Largest and smallest digit of a given number.
Next: Sum of the digits in each number in a list is equal.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.