Python: Reverse all the words which have odd length
Reverse Odd-Length Words
Write a Python program to reverse all words of odd lengths.
Sample Solution-1:
Python Code:
# Define a function 'test' that takes a string as input.
def test(txt):
# Use a generator expression within 'join' to reverse words with odd length and keep others unchanged.
return ' '.join(i[::-1] if len(i) % 2 else i for i in txt.split())
# Test case 1
text1 = "The quick brown fox jumps over the lazy dog"
print("Original string:")
print(text1)
print("Reverse all the words of the said string which have odd length:")
print(test(text1))
# Test case 2
text2 = "Python Exercises"
print("\nOriginal string:")
print(text2)
print("Reverse all the words of the said string which have odd length:")
print(test(text2))
Sample Output:
Original string: The quick brown fox jumps over the lazy dog Reverse all the words of the said string which have odd length: ehT kciuq nworb xof spmuj over eht lazy god Original string: Python Exercises Reverse all the words of the said string which have odd length: Python sesicrexE
Explanation:
Here is a breakdown of the above Python code:
- test Function:
- The "test()" function takes a string as input.
- It uses a generator expression within the "join" method to iterate over the words of the string.
- For each word, if the length of the word is odd, it reverses the word (i[::-1]), otherwise, it keeps the word unchanged (i).
Flowchart:
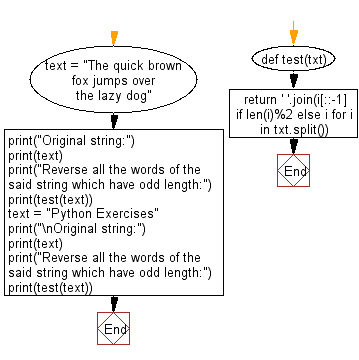
Sample Solution-2:
Python Code:
# Define a function 'test' that takes a string as input.
def test(text):
# Split the input string into a list of words using space as the delimiter.
result = list(text.split(' '))
# Iterate through each word in the list.
for i in range(len(result)):
# Check if the length of the current word is odd.
if len(result[i]) % 2 == 1:
# If the length is odd, reverse the word.
result[i] = result[i][::-1]
# Join the modified words back into a string using space as the separator.
return ' '.join(result)
# Test case 1
text1 = "The quick brown fox jumps over the lazy dog"
print("Original string:")
print(text1)
print("Reverse all the words of the said string which have odd length:")
print(test(text1))
# Test case 2
text2 = "Python Exercises"
print("\nOriginal string:")
print(text2)
print("Reverse all the words of the said string which have odd length:")
print(test(text2))
Sample Output:
Original string: The quick brown fox jumps over the lazy dog Reverse all the words of the said string which have odd length: ehT kciuq nworb xof spmuj over eht lazy god Original string: Python Exercises Reverse all the words of the said string which have odd length: Python sesicrexE
Flowchart:
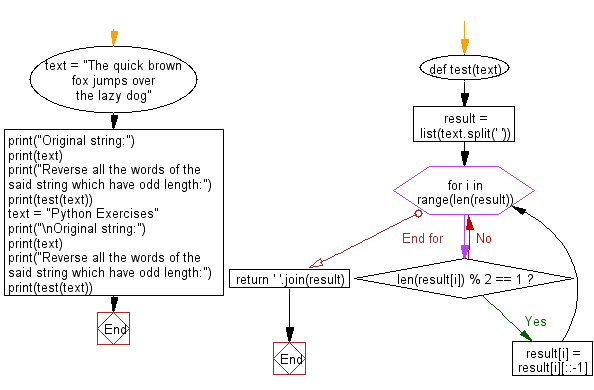
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to get the Least Common Multiple (LCM) of more than two numbers. Take the numbers from a given list of positive integers.
Next: Write a Python program to find the longest common ending between two given strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics