Python: Reverse a given string in lower case
Reverse String in Lowercase
Write a Python program to reverse a given string in lower case.
Sample Solution-1:
Python Code:
# Define a function 'test' that reverses the input string and converts it to lowercase.
def test(input_str):
# Using string slicing [::-1] to reverse the input string and lower() to convert it to lowercase.
return input_str[::-1].lower()
# Test the 'test' function with different input strings.
str1 = "PHP"
print("Original string:", str1)
print("Reverse the said string in lower case:", test(str1))
str2 = "JavaScript"
print("\nOriginal string:", str2)
print("Reverse the said string in lower case:", test(str2))
str3 = "PHPP"
print("\nOriginal string:", str3)
print("Reverse the said string in lower case:", test(str3))
Sample Output:
Original string: PHP Reverse the said string in lower case: php Original string: JavaScript Reverse the said string in lower case: tpircsavaj Original string: PHPP Reverse the said string in lower case: pphp
Explanation:
Here is a breakdown of the above Python code:
- Test Function (test function):
- The "test()" function is defined to reverse the input string and convert it to lowercase.
- It uses string slicing ([::-1]) to reverse the input string and the lower() method to convert it to lowercase.
Flowchart:
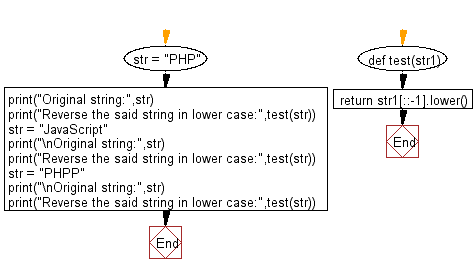
Sample Solution-2:
Python Code:
# Define a function 'test' that reverses the input string after converting it to lowercase.
def test(input_str):
# Using the lower() method to convert the input string to lowercase.
# Then, using string slicing [::-1] to reverse the lowercase input string.
return input_str.lower()[::-1]
# Test the 'test' function with different input strings.
str1 = "PHP"
print("Original string:", str1)
print("Reverse the said string in lower case:", test(str1))
str2 = "JavaScript"
print("\nOriginal string:", str2)
print("Reverse the said string in lower case:", test(str2))
str3 = "PHPP"
print("\nOriginal string:", str3)
print("Reverse the said string in lower case:", test(str3))
Sample Output:
Original string: PHP Reverse the said string in lower case: php Original string: JavaScript Reverse the said string in lower case: tpircsavaj Original string: PHPP Reverse the said string in lower case: pphp
Explanation:
Here is a breakdown of the above Python code:
- Test Function (test function):
- The "test()" function is defined to convert the input string to lowercase using lower() and then reverse it using string slicing ([::-1]).
Flowchart:
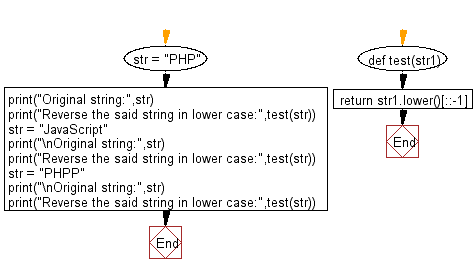
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to check if a given string contains two similar consecutive letters.
Next: Write a Python program to convert the letters of a given string (same case-upper/lower) into alphabetical order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics