Python: Create all possible permutations from a given collection of distinct numbers
List Permutations Generator
Write a Python program that generates a list of all possible permutations from a given collection of distinct numbers.
Visual Presentation:
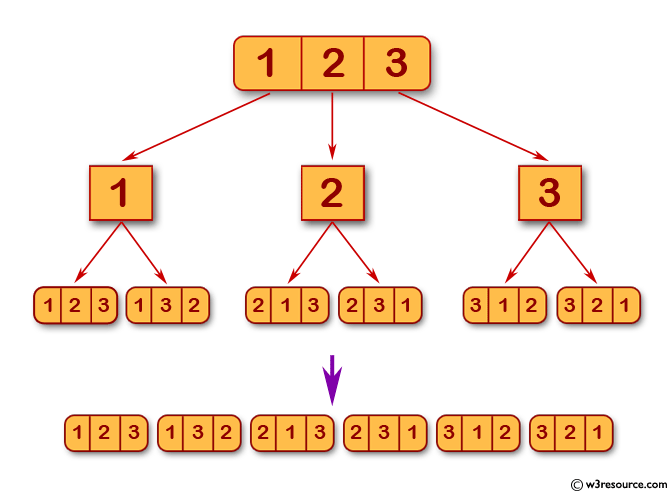
Sample Solution:
Python Code:
# Define a function 'permute' that generates all permutations of a list of numbers.
def permute(nums):
# Initialize the result list with an empty permutation.
result_perms = [[]]
# Iterate through each number in the input list.
for n in nums:
# Create a new list to store permutations with the current number.
new_perms = []
# Iterate through each existing permutation in the result list.
for perm in result_perms:
# Insert the current number at different positions within each existing permutation.
for i in range(len(perm)+1):
new_perms.append(perm[:i] + [n] + perm[i:])
# Update the result list with the new permutations.
result_perms = new_perms
# Return the final list of permutations.
return result_perms
# Create a list of numbers.
my_nums = [1, 2, 3]
# Print the original collection and the collection of distinct numbers.
print("Original Collection: ", my_nums)
print("Collection of distinct numbers:\n", permute(my_nums))
Sample Output:
Original Cofllection: [1, 2, 3] Collection of distinct numbers: [[3, 2, 1], [2, 3, 1], [2, 1, 3], [3, 1, 2], [1, 3, 2], [1, 2, 3]]
Explanation:
This above Python code defines a function called "permute()" that generates all permutations of a list of numbers. Here's a brief explanation:
- The function "permute()" takes a list of numbers ('nums') as input.
- It initializes a list 'result_perms' with an empty permutation to store the final results.
- It iterates through each number in the input list.
- For each number, it creates a new list ('new_perms') to store permutations with the current number.
- It iterates through each existing permutation in the result list.
- For each existing permutation, it inserts the current number at different positions to create new permutations.
- It updates the 'result_perms' list with the new permutations.
- The function returns the final list of permutations.
Flowchart:
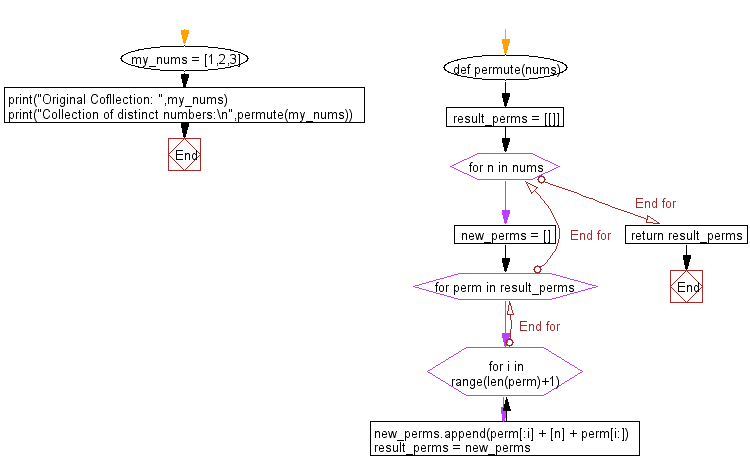
For more Practice: Solve these Related Problems:
- Write a Python program to generate all permutations of a list of numbers where repetition is allowed.
- Write a Python program to generate all permutations of the characters in a given string.
- Write a Python program to generate all partial permutations (of length k) from a list of distinct numbers.
- Write a Python program to compute the next lexicographic permutation for a given list of numbers.
Go to:
Previous: Write a Python program to check the sum of three elements (each from an array) from three arrays is equal to a target value. Print all those three-element combinations.
Next: Write a Python program to get all possible two digit letter combinations from a digit (1 to 9) string.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.