Python: Identify nonprime numbers between 1 to 100
Find Non-Prime Numbers
Write a Python program to identify non-prime numbers between 1 and 100 (integers). Print the non-prime numbers.
Sample Solution:
Python Code:
# Import the 'math' module to use mathematical functions.
import math
# Define a function 'is_not_prime' to check if a number is not prime.
def is_not_prime(n):
# Initialize the answer as False.
ans = False
# Iterate through potential divisors from 2 to the square root of 'n' (inclusive).
for i in range(2, int(math.sqrt(n)) + 1):
# If 'n' is divisible by 'i', set the answer to True.
if n % i == 0:
ans = True
# Return the final answer.
return ans
# Print a message indicating the purpose of the following loop.
print("Nonprime numbers between 1 to 100:")
# Use the 'filter' function to create an iterable of nonprime numbers.
# Iterate through the filtered numbers and print each one.
for x in filter(is_not_prime, range(1, 101)):
print(x)
Sample Output:
Nonprime numbers between 1 to 100: 4 6 8 9 10 12 14 15 .. 90 91 92 93 94 95 96 98 99 100
Explanation:
Here is a breakdown of the above Python code:
- Module Import:
- The code imports the "math" module to use mathematical functions, specifically, the square root function.
- Function definition - is_not_prime:
- A function named "is_not_prime()" is defined to check if a given number is not prime.
- The function uses a loop to iterate through potential divisors and sets the answer to True if any divisor is found.
- Printing message:
- A message is printed to indicate the purpose of the following loop.
- Filtering and printing nonprime numbers:
- The "filter()" function is used to create an iterable of nonprime numbers in the range from 1 to 100.
- The iterable is then looped through, and each nonprime number is printed.
Flowchart:
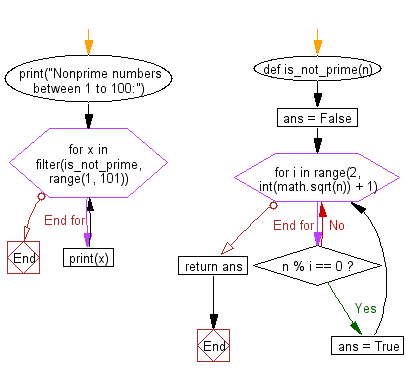
For more Practice: Solve these Related Problems:
- Write a Python program to list all composite numbers between 1 and 100 by checking for non-prime conditions.
- Write a Python program to generate non-prime numbers within a specified range by testing for factors.
- Write a Python program to filter out prime numbers from a range and output the remaining composite numbers.
- Write a Python program to compute and print all non-prime integers between 1 and 100 using a helper function.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to generate and prints a list of numbers from 1 to 10.
Next: Write a Python program to make a request to a web page, and test the status code, also display the html code of the specified web page.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics