Python: Find the indices of all occurrences of a given item in a given list
Find Indices of Item
Write a Python program to find the indices of all occurrences of a given item in a given list.
Sample Solution-1:
Python Code:
# Define a function named indices_in_list that returns a list of indices where a given item (n) occurs in a list (nums_list).
def indices_in_list(nums_list, n):
# Use list comprehension to create a list of indices where the item equals n.
return [idx for idx, i in enumerate(nums_list) if i == n]
# Test the function with different lists and items, and print the results.
# Test case 1
nums = [1,2,3,4,5,2]
print("Original list of numbers:", nums)
n = 2
print("Given Number:", n)
print("Indices of all occurrences of the said item in the given list:")
print(indices_in_list(nums, n))
# Test case 2
nums = [3,1,2,3,4,5,6,3,3]
print("\nOriginal list of numbers:", nums)
n = 3
print("Given Number:", n)
print("Indices of all occurrences of the said item in the given list:")
print(indices_in_list(nums, n))
# Test case 3
nums = [1,2,3,-4,5,2,-4]
print("\nOriginal list of numbers:", nums)
n = -4
print("Given Number:", n)
print("Indices of all occurrences of the said item in the given list:")
print(indices_in_list(nums, n))
# Test case 4
nums = [1,2,3,4,5,2]
print("\nOriginal list of numbers:", nums)
n = 7
print("Given Number:", n)
print("Indices of all occurrences of the said item in the given list:")
print(indices_in_list(nums, n))
Sample Output:
Original list of numbers: [1, 2, 3, 4, 5, 2] Given Number 2 Indices of all occurrences of the said item in the given list: [1, 5] Original list of numbers: [3, 1, 2, 3, 4, 5, 6, 3, 3] Given Number 3 Indices of all occurrences of the said item in the given list: [0, 3, 7, 8] Original list of numbers: [1, 2, 3, -4, 5, 2, -4] Given Number -4 Indices of all occurrences of the said item in the given list: [3, 6] Original list of numbers: [1, 2, 3, 4, 5, 2] Given Number 7 Indices of all occurrences of the said item in the given list: []
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "indices_in_list()" that returns a list of indices where a given item (n) occurs in a list (nums_list).
- List comprehension:
- The function uses list comprehension to iterate through the elements of 'nums_list' along with their indices and creates a list of indices where the element is equal to 'n'.
- Test cases:
- The function is tested with different lists and items using print(indices_in_list(...)).
Visual Presentation:
Flowchart:
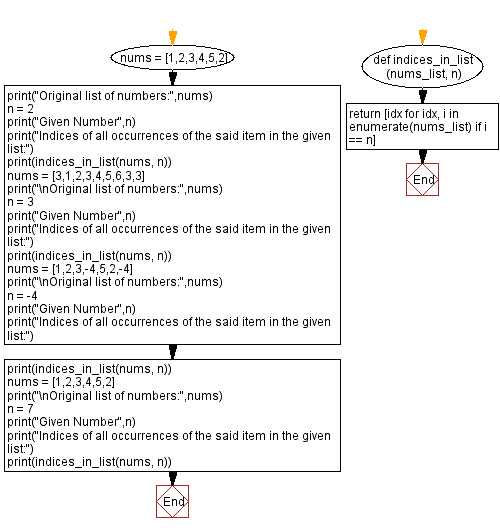
For more Practice: Solve these Related Problems:
- Write a Python program to find all indices where a given element appears in a list using the enumerate function.
- Write a Python program to return a list of indices for every occurrence of a target value in an array.
- Write a Python program to iterate through a list and record the positions of a specified item using list comprehension.
- Write a Python program to search for a given value in a list and output its indices without using built-in functions.
Sample Solution-2:
Python Code:
# Import the count function from the itertools module
from itertools import count
# Define a function named indices_in_list that returns a list of indices where a given item (n) occurs in a list (nums_list).
def indices_in_list(nums_list, n):
# Use list comprehension to create a list of indices where the item equals n, using the count() function to generate indices.
return [(i) for i, j in zip(count(), nums_list) if j == n]
# Test the function with different lists and items, and print the results.
# Test case 1
nums = [1,2,3,4,5,2]
print("Original list of numbers:", nums)
n = 2
print("Given Number:", n)
print("Indices of all occurrences of the said item in the given list:")
print(indices_in_list(nums, n))
# Test case 2
nums = [3,1,2,3,4,5,6,3,3]
print("\nOriginal list of numbers:", nums)
n = 3
print("Given Number:", n)
print("Indices of all occurrences of the said item in the given list:")
print(indices_in_list(nums, n))
# Test case 3
nums = [1,2,3,-4,5,2,-4]
print("\nOriginal list of numbers:", nums)
n = -4
print("Given Number:", n)
print("Indices of all occurrences of the said item in the given list:")
print(indices_in_list(nums, n))
# Test case 4
nums = [1,2,3,4,5,2]
print("\nOriginal list of numbers:", nums)
n = 7
print("Given Number:", n)
print("Indices of all occurrences of the said item in the given list:")
print(indices_in_list(nums, n))
Sample Output:
Original list of numbers: [1, 2, 3, 4, 5, 2] Given Number 2 Indices of all occurrences of the said item in the given list: [1, 5] Original list of numbers: [3, 1, 2, 3, 4, 5, 6, 3, 3] Given Number 3 Indices of all occurrences of the said item in the given list: [0, 3, 7, 8] Original list of numbers: [1, 2, 3, -4, 5, 2, -4] Given Number -4 Indices of all occurrences of the said item in the given list: [3, 6] Original list of numbers: [1, 2, 3, 4, 5, 2] Given Number 7 Indices of all occurrences of the said item in the given list: []
Explanation:
Here is a breakdown of the above Python code:
- Import statement:
- The code imports the count function from the itertools module.
- Function definition:
- The code defines a function named indices_in_list that returns a list of indices where a given item (n) occurs in a list (nums_list).
- List comprehension with count():
- The function uses list comprehension to iterate through the elements of nums_list along with their indices generated by count(), and creates a list of indices where the element is equal to n.
- Test cases:
- The function is tested with different lists and items using print(indices_in_list(...)).
Flowchart:
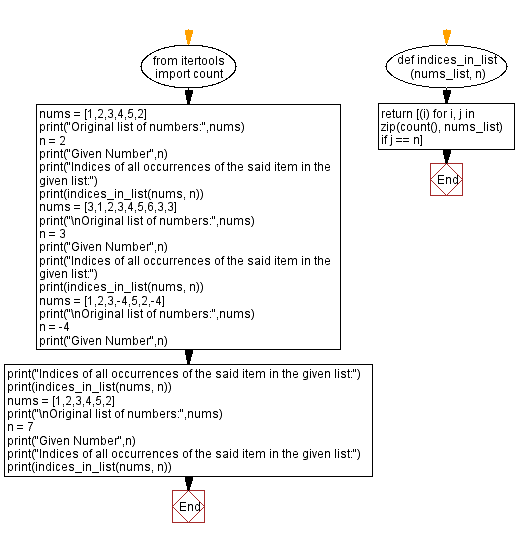
Sample Solution-3:
Python Code:
# Import the locate function from the more_itertools module
from more_itertools import locate
# Define a function named indices_in_list that returns a list of indices where a given item (n) occurs in a list (nums_list).
def indices_in_list(nums_list, n):
# Use the locate function to find all indices where the item equals n and convert it to a list.
return list(locate(nums_list, lambda x: x == n))
# Test the function with different lists and items, and print the results.
# Test case 1
nums = [1,2,3,4,5,2]
print("Original list of numbers:", nums)
n = 2
print("Given Number:", n)
print("Indices of all occurrences of the said item in the given list:")
print(indices_in_list(nums, n))
# Test case 2
nums = [3,1,2,3,4,5,6,3,3]
print("\nOriginal list of numbers:", nums)
n = 3
print("Given Number:", n)
print("Indices of all occurrences of the said item in the given list:")
print(indices_in_list(nums, n))
# Test case 3
nums = [1,2,3,-4,5,2,-4]
print("\nOriginal list of numbers:", nums)
n = -4
print("Given Number:", n)
print("Indices of all occurrences of the said item in the given list:")
print(indices_in_list(nums, n))
# Test case 4
nums = [1,2,3,4,5,2]
print("\nOriginal list of numbers:", nums)
n = 7
print("Given Number:", n)
print("Indices of all occurrences of the said item in the given list:")
print(indices_in_list(nums, n))
Sample Output:
Original list of numbers: [1, 2, 3, 4, 5, 2] Given Number 2 Indices of all occurrences of the said item in the given list: [1, 5] Original list of numbers: [3, 1, 2, 3, 4, 5, 6, 3, 3] Given Number 3 Indices of all occurrences of the said item in the given list: [0, 3, 7, 8] Original list of numbers: [1, 2, 3, -4, 5, 2, -4] Given Number -4 Indices of all occurrences of the said item in the given list: [3, 6] Original list of numbers: [1, 2, 3, 4, 5, 2] Given Number 7 Indices of all occurrences of the said item in the given list: []
Explanation:
Here is a breakdown of the above Python code:
- Import statement:
- The code imports the "locate" function from the "more_itertools()" module.
- Function definition:
- The code defines a function named "indices_in_list()" that returns a list of indices where a given item (n) occurs in a list (nums_list).
- Use of locate function:
- The "locate()" function is used to find all indices where the element equals n, and the result is converted to a list.
- Test cases:
- The function is tested with different lists and items using print(indices_in_list(...)).
Flowchart:
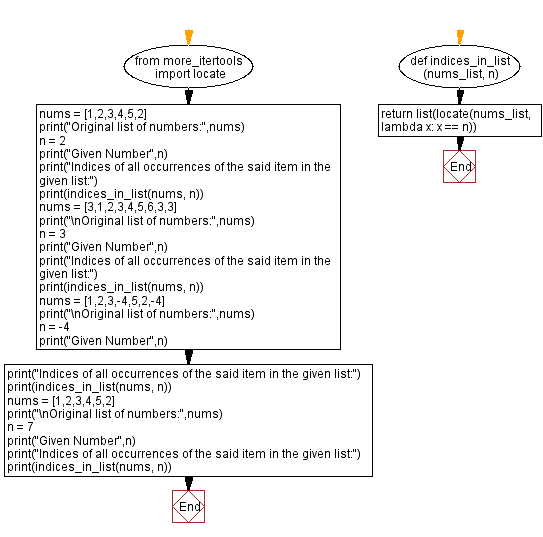
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous:Write a Python program that takes three integers and check whether the sum of the last digit of first number and the last digit of second number equal to the last digit of third number.
Next: Write a Python program to remove the duplicate numbers from a given list of numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics