Python: Sum of the last digit of first number and the last digit of second number equal to the last digit of third number
Sum of Last Digits
Write a Python program that takes three integers and checks whether the sum of the last digit of the first number and the last digit of the second number equals the last digit of the third number.
Sample Solution:
Python Code:
# Define a function named check_last_digit that checks if the last digits of the sum of x and z are equal to the last digit of y.
def check_last_digit(x, y, z):
# Convert the sum of x and z to a string, extract the last digit, and compare it with the last digit of y.
return str(x + z)[-1] == str(y)[-1]
# Test the function with different values for x, y, and z and print the results.
# Test case 1
print(check_last_digit(12, 26, 44))
# Test case 2
print(check_last_digit(145, 122, 1010))
# Test case 3
print(check_last_digit(0, 20, 40))
# Test case 4
print(check_last_digit(1, 22, 40))
# Test case 5
print(check_last_digit(145, 129, 104))
Sample Output:
True False True False True
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "check_last_digit()" that checks if the last digits of the sum of 'x' and 'z' are equal to the last digit of 'y'.
- String Conversion and Last Digit Extraction:
- The function converts the sum of 'x' and 'z' to a string, extracts the last digit using [-1], and compares it with the last digit of 'y'.
Pictorial Presentation:
Flowchart:
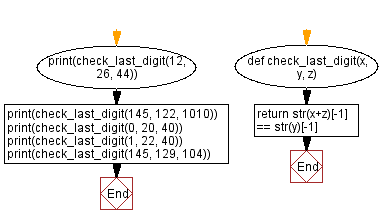
For more Practice: Solve these Related Problems:
- Write a Python program to check if the sum of the last digits of two numbers equals the last digit of a third number using modular arithmetic.
- Write a Python program to extract the last digit from two integers and compare their sum with the last digit of another integer.
- Write a Python program to use string slicing to obtain the last digit of numbers and verify if their sum matches the third number's last digit.
- Write a Python program to implement a function that compares the sum of two numbers' last digits to the last digit of a third number.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to check whether a given number is Oddish or Evenish.
Next: Write a Python program find the indices of all occurrences of a given item in a given list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.