Python: Check whether a given number is Oddish or Evenish
Oddish or Evenish
Write a Python program to check whether a given number is odd or even.
A number is called "Oddish" if the sum of all of its digits is odd, and a number is called "Evenish" if the sum of all of its digits is even.
Sample Solution:
Python Code:
# Define a function named oddish_evenish_num that checks whether the sum of digits of a number (n) is odd or even.
def oddish_evenish_num(n):
# Use the sum function to calculate the sum of all digits in the number.
# Check if the sum is odd and return 'Oddish', otherwise return 'Evenish'.
return 'Oddish' if sum(map(int, str(n))) % 2 else 'Evenish'
# Test the function with different numbers and print the results.
# Test case 1
n = 120
print("Original Number:", n)
print("Check whether the sum of all digits of the said number is odd or even!")
print(oddish_evenish_num(120))
# Test case 2
n = 321
print("\nOriginal Number:", n)
print("Check whether the sum of all digits of the said number is odd or even!")
print(oddish_evenish_num(321))
# Test case 3
n = 43
print("\nOriginal Number:", n)
print("Check whether the sum of all digits of the said number is odd or even!")
print(oddish_evenish_num(43))
# Test case 4
n = 4433
print("\nOriginal Number:", n)
print("Check whether the sum of all digits of the said number is odd or even!")
print(oddish_evenish_num(4433))
# Test case 5
n = 373
print("\nOriginal Number:", n)
print("Check whether the sum of all digits of the said number is odd or even!")
print(oddish_evenish_num(373))
Sample Output:
Original Number 120 Check whether the sum of all digits of the said number is odd or even! Oddish Original Number 321 Check whether the sum of all digits of the said number is odd or even! Evenish Original Number 43 Check whether the sum of all digits of the said number is odd or even! Oddish Original Number 4433 Check whether the sum of all digits of the said number is odd or even! Evenish Original Number 373 Check whether the sum of all digits of the said number is odd or even! Oddish
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "oddish_evenish_num()" that checks whether the sum of digits of a given number (n) is odd or even.
- Sum of Digits:
- The sum(map(int, str(n))) expression converts the number to a string, maps each character to its integer equivalent, and calculates the sum of these integers.
- Oddish or Evenish Check:
- The function checks if the sum is odd using % 2 and returns 'Oddish' if true, otherwise 'Evenish'.
Visual Presentation:
Flowchart:
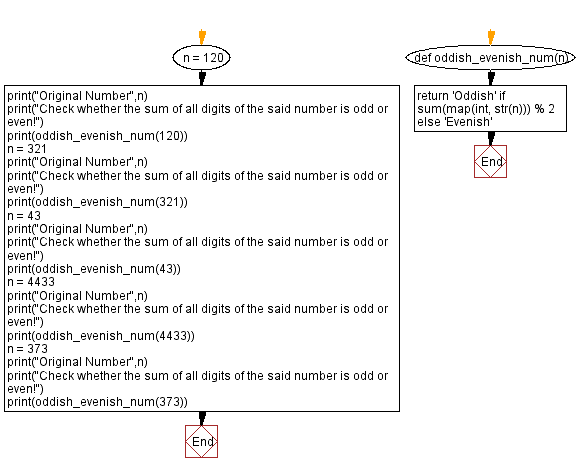
For more Practice: Solve these Related Problems:
- Write a Python program to classify a number as "Oddish" if the sum of its digits is odd and "Evenish" if the sum is even.
- Write a Python program to compute the sum of digits of a number and output "Oddish" or "Evenish" based on its parity.
- Write a Python program to determine if a number is Oddish or Evenish by converting it to a string and summing its digits.
- Write a Python program to implement a function that returns "Oddish" or "Evenish" after computing the digit sum of a number.
Go to:
Previous: Write a Python program to test whether a given integer is Pandigital number or not.
Next: Write a Python program that takes three integers and check whether the sum of the last digit of first number and the last digit of second number equal to the last digit of third number.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.