PostgreSQL Basic SELECT Statement: Display the employee ID, name and salary in ascending order according to salary
5. Write a query to get the employee ID, name (first_name, last_name) and salary in ascending order according to their salary.
Sample Solution:
Code:
-- Selecting employee_id, first_name, last_name, and salary from the employees table
SELECT employee_id, first_name, last_name, salary
-- Ordering the result set by the salary column in ascending order
FROM employees
ORDER BY salary;
Explanation:
- The SELECT statement is used to retrieve data from a database table.
- It selects the columns employee_id, first_name, last_name, and salary from the employees table.
- The ORDER BY clause is used to sort the results based on the salary column in ascending order.
- This query retrieves the employee_id, first_name, last_name, and salary of each employee from the employees table, ordered by their salary in ascending order.
Sample table: employees
Output:
pg_exercises=# SELECT employee_id, first_name, last_name, salary pg_exercises-# FROM employees pg_exercises-# ORDER BY salary; employee_id | first_name | last_name | salary -------------+-------------+-------------+---------- 132 | TJ | Olson | 2100.00 128 | Steven | Markle | 2200.00 136 | Hazel | Philtanker | 2200.00 135 | Ki | Gee | 2400.00 127 | James | Landry | 2400.00 140 | Joshua | Patel | 2500.00 119 | Karen | Colmenares | 2500.00 182 | Martha | Sullivan | 2500.00 144 | Peter | Vargas | 2500.00 131 | James | Marlow | 2500.00 191 | Randall | Perkins | 2500.00 143 | Randall | Matos | 2600.00 199 | Douglas | Grant | 2600.00 118 | Guy | Himuro | 2600.00 198 | Donald | OConnell | 2600.00 126 | Irene | Mikkilineni | 2700.00 139 | John | Seo | 2700.00 183 | Girard | Geoni | 2800.00 195 | Vance | Jones | 2800.00 ...
Relational Algebra Expression:

Relational Algebra Tree:
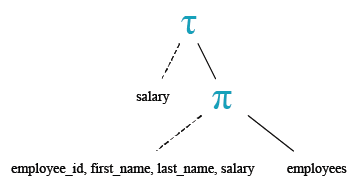
Practice Online
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a query to get the names (first_name, last_name), salary and 15% of salary as PF for all the employees.
Next: Write a query to get the total salaries payable to employees.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics