Math operations package in PL/SQL
PL/SQL Package: Exercise-4 with Solution
Write a PL/SQL code that implement a package that includes procedures and functions to perform complex mathematical operations, such as finding the nth root of a number and calculating logarithms.
Sample Solution:
PL/SQL Code:
CREATE OR REPLACE PACKAGE MathOperationsPackage AS
PROCEDURE NthRoot(input_number IN NUMBER, n IN NUMBER, result OUT NUMBER);
FUNCTION Logarithm(input_number IN NUMBER, base IN NUMBER) RETURN NUMBER;
END MathOperationsPackage;
/
CREATE OR REPLACE PACKAGE BODY MathOperationsPackage AS
PROCEDURE NthRoot(input_number IN NUMBER, n IN NUMBER, result OUT NUMBER) IS
BEGIN
IF input_number< 0 AND n MOD 2 = 0 THEN
RAISE_APPLICATION_ERROR(-20001, 'Cannot calculate even root of a negative number.');
END IF;
result := input_number**(1/n);
EXCEPTION
WHEN OTHERS THEN
RAISE_APPLICATION_ERROR(-20002, 'An error occurred while calculating the nth root.');
END NthRoot;
FUNCTION Logarithm(input_number IN NUMBER, base IN NUMBER) RETURN NUMBER IS
BEGIN
IF input_number<= 0 OR base <= 0 THEN
RAISE_APPLICATION_ERROR(-20003, 'Both input number and base must be greater than zero.');
END IF;
RETURN LOG(input_number, base);
EXCEPTION
WHEN OTHERS THEN
RAISE_APPLICATION_ERROR(-20004, 'An error occurred while calculating the logarithm.');
END Logarithm;
END MathOperationsPackage;
/
Sample Output:
Package created. Package Body created.
Flowchart:
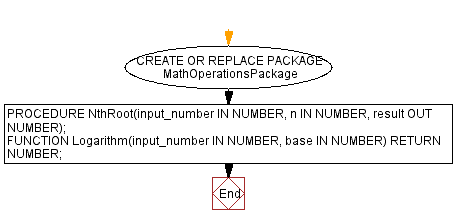
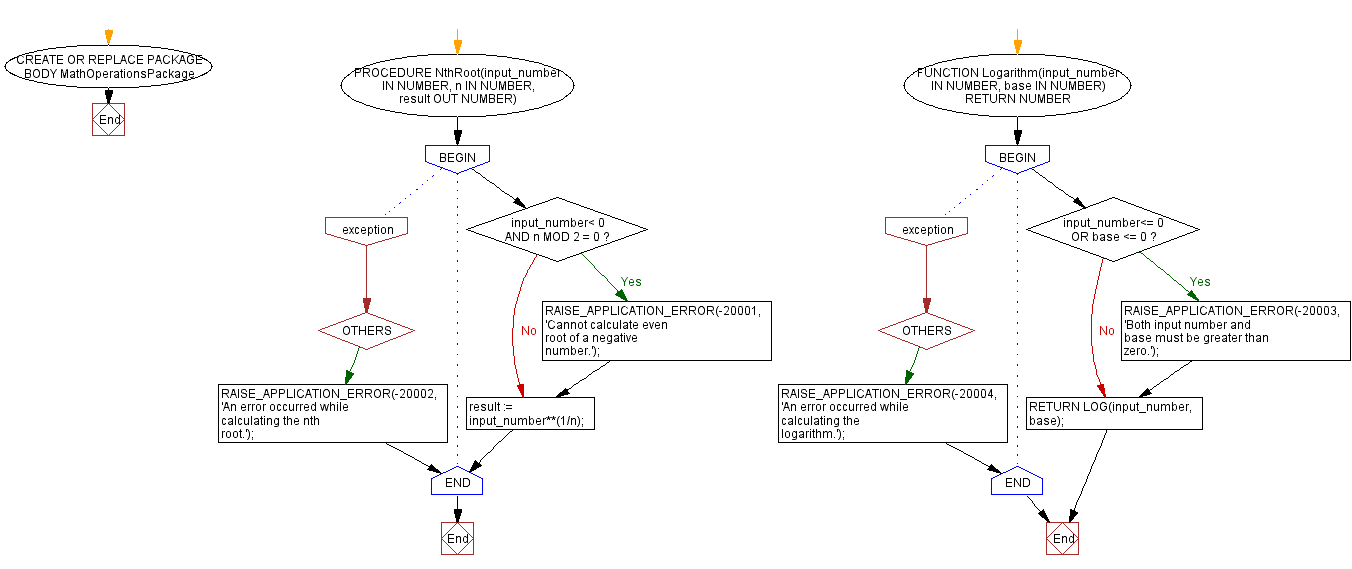
To execute the package:
DECLARE
root_result NUMBER;
logarithm_result NUMBER;
BEGIN
MathOperationsPackage.NthRoot(16, 2, root_result);
DBMS_OUTPUT.PUT_LINE('Square root of 16: ' || root_result);
MathOperationsPackage.NthRoot(-8, 3, root_result);
DBMS_OUTPUT.PUT_LINE('Cube root of -8: ' || root_result);
EXCEPTION
WHEN OTHERS THEN
DBMS_OUTPUT.PUT_LINE(SQLERRM);
END;
/
Sample Output:
Statement processed. Square root of 16: 4 ORA-20002: An error occurred while calculating the nth root.
Flowchart:
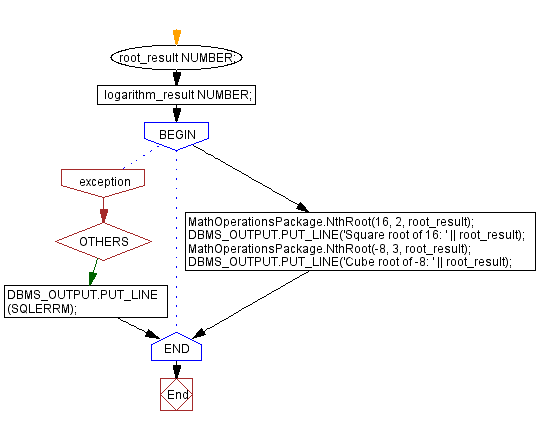
Explanation:
The said code in Oracle's PL/SQL package that provides a convenient way to perform mathematical operations like nth root and logarithm in PL/SQL programs while ensuring the validity of input parameters and handling potential errors.
The PL/SQL package that includes two procedures "NthRoot" and "Logarithm".
The "NthRoot" procedure accepts the parameters "input_number", "n", and "result". It first checks whether the input_number is negative and the root value is even. If satisfied both, raises an application error with a message "even root of a negative number cannot be calculated". Otherwise, it performs the nth root calculation and assigns the result to the "result" output parameter.
The "Logarithm" function takes the parameters "input_number" and "base". It checks whether both the input_number and base are greater than zero or not. An application error raises If either of them is zero or negative, with a message both the input number and base must be greater than zero. The function then calculates the logarithm and returns the result.
Previous: Input validation package in PL/SQL.
Next: PL/SQL package for password generation and strength checking.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics