MathUtils Package - Factorial Calculation and Prime Number Check
PL/SQL Package: Exercise-1 with Solution
Write a PL/SQL code to create a package that includes a procedure to calculate the factorial of a number and a function to check if a number is prime.
Sample Solution:
PL/SQL Code:
CREATE OR REPLACE PACKAGE MathUtils IS
PROCEDURE CalculateFactorial(n IN NUMBER, result OUT NUMBER);
FUNCTION IsPrime(n IN NUMBER) RETURN BOOLEAN;
END MathUtils;
/
CREATE OR REPLACE PACKAGE BODY MathUtils IS
PROCEDURE CalculateFactorial(n IN NUMBER, result OUT NUMBER) IS
factorial NUMBER := 1;
BEGIN
IF n < 0 THEN
RAISE_APPLICATION_ERROR(-20001, 'Factorial is not defined for negative numbers.');
END IF;
IF n > 1 THEN
FOR i IN 2..n LOOP
factorial := factorial * i;
END LOOP;
END IF;
result := factorial;
END CalculateFactorial;
FUNCTION IsPrime(n IN NUMBER) RETURN BOOLEAN IS
divisor NUMBER := 2;
BEGIN
IF n < 2 THEN
RETURN FALSE;
END IF;
WHILE divisor <= SQRT(n) LOOP
IF n MOD divisor = 0 THEN
RETURN FALSE;
END IF;
divisor := divisor + 1;
END LOOP;
RETURN TRUE;
END IsPrime;
END MathUtils;
/
Sample Output:
Package created. Package Body created.
Flowchart:
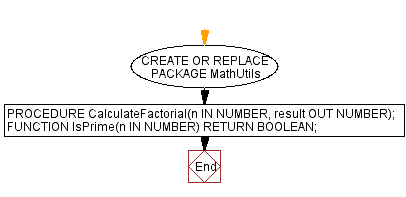
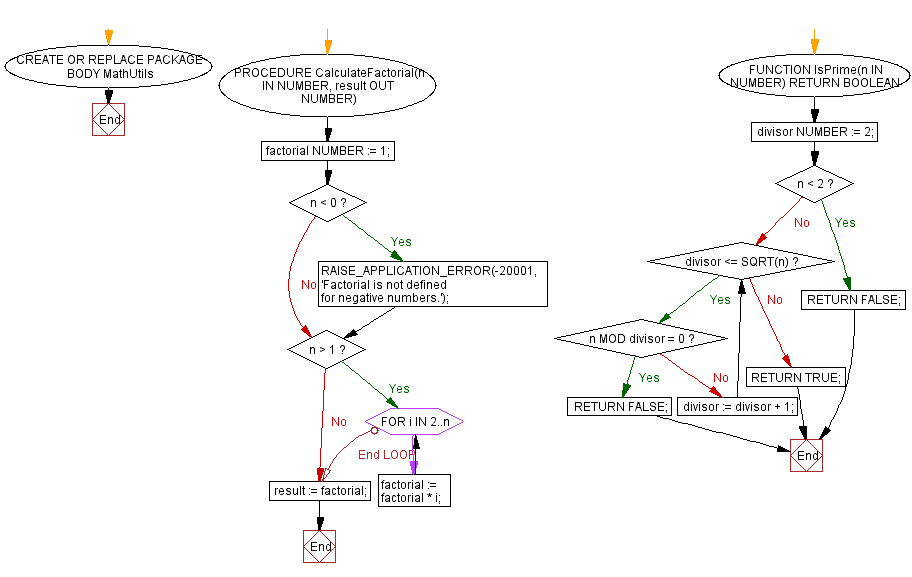
To execute the package:
DECLARE
factorial_result NUMBER;
is_prime_result BOOLEAN;
BEGIN
MathUtils.CalculateFactorial(7, factorial_result);
DBMS_OUTPUT.PUT_LINE('Factorial of 7: ' || factorial_result);
is_prime_result := MathUtils.IsPrime(7);
IF is_prime_result THEN
DBMS_OUTPUT.PUT_LINE('7 is prime.');
ELSE
DBMS_OUTPUT.PUT_LINE('7 is not prime.');
END IF;
END;
/
Sample Output:
Statement processed. Factorial of 7: 5040 7 is prime.
Flowchart:
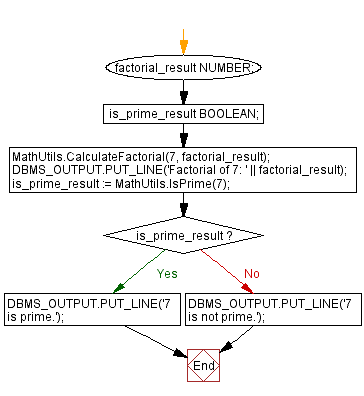
Explanation:
The said code in Oracle's PL/SQL package that provides convenient functions for factorial calculation and prime number checking in PL/SQL.
The two main functionalities calculating the factorial of a given number and checking if a number is prime are done in the code.
In CalculateFactorial Procedure an input parameter 'n' of type NUMBER and an output parameter 'result' of type NUMBER declared. Using loop calculates the factorial of 'n' that iterates from 2 to 'n' and multiplies the current value of 'factorial' by 'i'. Raises an exception with a custom error message if 'n' is a negative number.
In IsPrime Function an input parameter 'n' of type NUMBER and returns a BOOLEAN value.
Checks if 'n' is less than 2 and returns FALSE, as numbers less than 2 are not prime.
Utilizes a loop that starts from 2 and iterates until the square root of 'n'.
Checks if 'n' is divisible by the current 'divisor' using the modulo operation.
If 'n' is divisible by any 'divisor', it returns FALSE, indicating that 'n' is not prime.
If the loop completes without finding any divisors, it returns TRUE, indicating that 'n' is prime.
Previous: PL/SQL Package Exercises Home.
Next: String Manipulation Functions.
What is the difficulty level of this exercise?