PL/SQL Method to Determine whether a Shape is a Square or not
PL/SQL OOP: Exercise-6 with Solution
Write a PL/SQL code that implement a method in the "Shape" class to determine if the shape is a square or not.
Sample Solution:
PL/SQL Code:
-- Define the Shape type with attributes representing sides and methods
CREATE TYPE Shape AS OBJECT
(
-- Attributes to represent the shape
side1 NUMBER,
side2 NUMBER,
side3 NUMBER,
side4 NUMBER,
-- Constructor to initialize the shape attributes
CONSTRUCTOR FUNCTION Shape (side1 NUMBER, side2 NUMBER, side3 NUMBER, side4 NUMBER) RETURN SELF AS RESULT,
-- Method to check if the shape is a square
MEMBER FUNCTION isSquare RETURN BOOLEAN
);
/
-- Define the body of the Shape type
CREATE TYPE BODY Shape AS
-- Constructor implementation
CONSTRUCTOR FUNCTION Shape (side1 NUMBER, side2 NUMBER, side3 NUMBER, side4 NUMBER) RETURN SELF AS RESULT IS
BEGIN
-- Initialize the attributes with provided values
SELF.side1 := side1;
SELF.side2 := side2;
SELF.side3 := side3;
SELF.side4 := side4;
RETURN;
END;
-- Method implementation to check if the shape is a square
MEMBER FUNCTION isSquare RETURN BOOLEAN IS
BEGIN
-- Check if all sides are equal, indicating a square
IF side1 = side2 AND side1 = side3 AND side1 = side4 THEN
RETURN TRUE;
ELSE
RETURN FALSE;
END IF;
END;
END;
/
Now the following code is the instances of the class "Shape" with the "isSquare" method, and check whether they represent squares or not.
PL/SQL Code:
-- Declare and initialize instances of the Shape type
DECLARE
-- Creating a rectangle (not a square)
rect Shape := Shape(5, 10, 5, 10);
-- Creating a square
square Shape := Shape(3, 3, 3, 3);
BEGIN
-- Checking if the rectangle is a square
IF rect.isSquare THEN
DBMS_OUTPUT.PUT_LINE('The rectangle is a square.');
ELSE
DBMS_OUTPUT.PUT_LINE('The rectangle is not a square.');
END IF;
-- Checking if the square is a square
IF square.isSquare THEN
DBMS_OUTPUT.PUT_LINE('The square is a square.');
ELSE
DBMS_OUTPUT.PUT_LINE('The square is not a square.');
END IF;
END;
Sample Output:
Statement processed. The rectangle is not a square. The square is a square.
Flowchart:
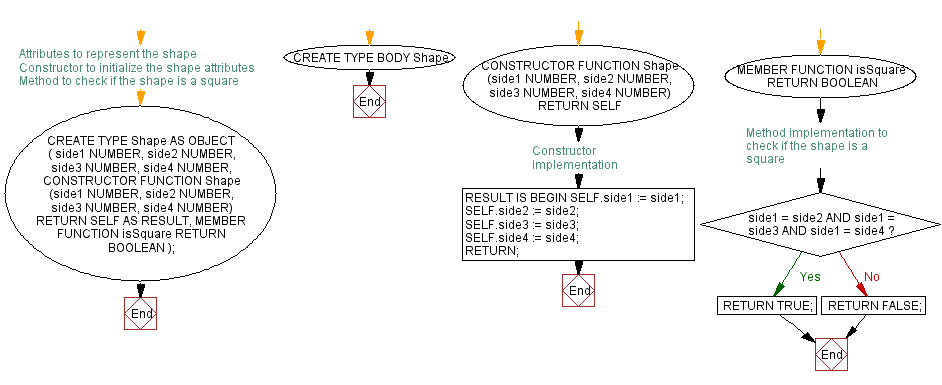
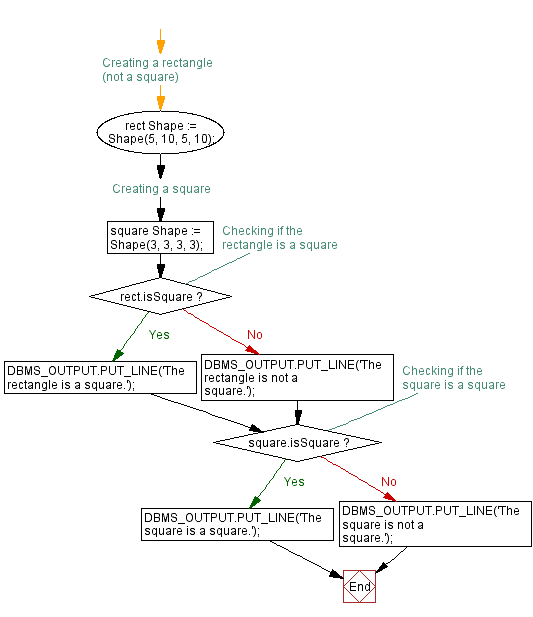
Explanation:
The said code in Oracle's PL/SQL that demonstrated how to create instances of the "Shape" class and use the "isSquare" method to determine if the shapes are squares or not.
The class "Shape" is declared that have attributes that describe the shape's properties, such as length, width, or sides and it contains a method "isSquare" to check whether the shape is a square or not.
For a shape to be considered a square, it needs to have equal length sides and right angles.
Previous: Creating a "Shape" Class in PL/SQL with Area Calculation Method.
What is the difficulty level of this exercise?