Creating a "Shape" Class in PL/SQL with Area Calculation Method
PL/SQL OOP: Exercise-5 with Solution
Write a PL/SQL code to create a "Shape" class with attributes like length and width, and implement a method to calculate the area.
Sample Solution:
PL/SQL Code:
-- Create the "Shape" class
CREATE OR REPLACE TYPE Shape AS OBJECT (
length NUMBER, -- Attribute for length
width NUMBER, -- Attribute for width
-- Method to calculate the area
MEMBER FUNCTION calculate_area RETURN NUMBER
);
/
Now create the body of the ‘Shape’ class
PL/SQL Code:
-- Create the body of the "Shape" class
CREATE OR REPLACE TYPE BODY Shape AS
-- Method implementation to calculate the area
MEMBER FUNCTION calculate_area RETURN NUMBER IS
BEGIN
-- Calculate the area based on length and width
RETURN self.length * self.width;
END;
END;
/
Explanation:
A class representing a "Shape" with attributes like length and width to represent the dimensions of the shape is defined which is used to implement the calculation the area of the shape based on its dimensions.
To create and manipulate "Shape" objects the below functionality is used:
PL/SQL Code:
-- Example of using the "Shape" class
DECLARE
my_shape Shape;
area NUMBER;
BEGIN
-- Create a "Shape" object
my_shape := Shape(10, 5); -- Shape with length = 10 and width = 5
-- Calculate the area using the method
area := my_shape.calculate_area;
-- Display the result
DBMS_OUTPUT.PUT_LINE('Area of the Shape: ' || area);
END;
/
Sample Output:
Statement processed. Area of the Shape: 50
Flowchart:
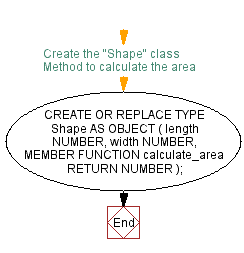
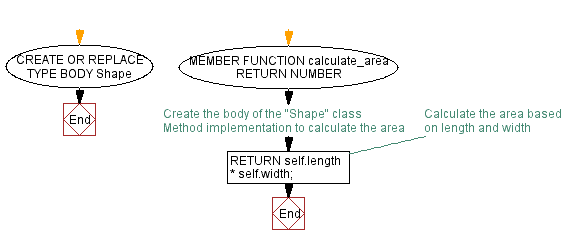
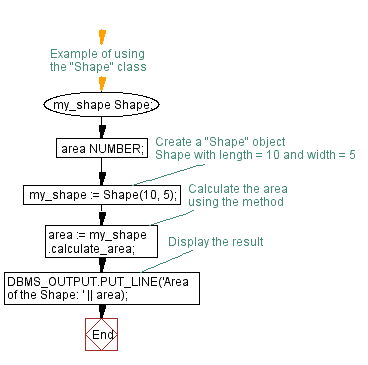
Explanation:
The said code in Oracle's PL/SQL that creating a "Shape" class with an area calculation method, it can be easily reuse this class to represent different shapes and perform area calculations based on their dimensions.
Previous: Creating a Subclass "Manager" in PL/SQL Inheriting from "Employee" Class.
Next: PL/SQL Method to Determine whether a Shape is a Square or not.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics