Method to calculate annual bonus based on salary in PL/SQL's "Employee" Class
PL/SQL OOP: Exercise-3 with Solution
Write a PL/SQL code to implement a method to calculate the annual bonus based on the salary in the "Employee" class.
Sample Solution:
PL/SQL Code:
-- Create the Employee class
CREATE TYPE Employee AS OBJECT (
emp_id NUMBER, -- Attribute for employee ID
emp_nameVARCHAR2(100), -- Attribute for employee name
salary NUMBER, -- Attribute for employee salary
-- Method to calculate annual bonus
MEMBER FUNCTION calculate_bonus RETURN NUMBER
);
-- Create the body of the Employee class
CREATE TYPE BODY Employee AS
-- Method implementation to calculate the annual bonus
MEMBER FUNCTION calculate_bonus RETURN NUMBER IS
bonus_percentage NUMBER;
bonus_amount NUMBER;
BEGIN
-- Determine the bonus percentage based on salary
IF self.salary< 50000 THEN
bonus_percentage := 0.1; -- 10% bonus for salaries below 50000
ELSIF self.salary< 100000 THEN
bonus_percentage := 0.15; -- 15% bonus for salaries between 50000 and 100000
ELSE
bonus_percentage := 0.2; -- 20% bonus for salaries above 100000
END IF;
-- Calculate the bonus amount
bonus_amount := self.salary * bonus_percentage;
-- Return the calculated bonus
RETURN bonus_amount;
END;
END;
/
In the above code, a class an "Employee" defines with attributes emp_id, emp_name, and salary and implements a method calculate_bonus to calculate the annual bonus determines the bonus percentage based on different salary ranges and then calculates the corresponding bonus amount.
To use this Employee objects:
PL/SQL Code:
-- Example of using the Employee class
DECLARE
emp_obj Employee; -- Declare an instance of the Employee class
emp_bonus NUMBER; -- Declare a variable to store the bonus amount
BEGIN
-- Create an Employee object with ID 1, name 'Musa Lara', and salary 75000
emp_obj := Employee(1, 'Musa Lara', 75000);
-- Calculate the annual bonus using the calculate_bonus method
emp_bonus := emp_obj.calculate_bonus;
-- Display the result
DBMS_OUTPUT.PUT_LINE('Employee Bonus: ' || emp_bonus);
END;
/
Sample Output:
Statement processed. Employee Bonus: 11250
Flowchart:
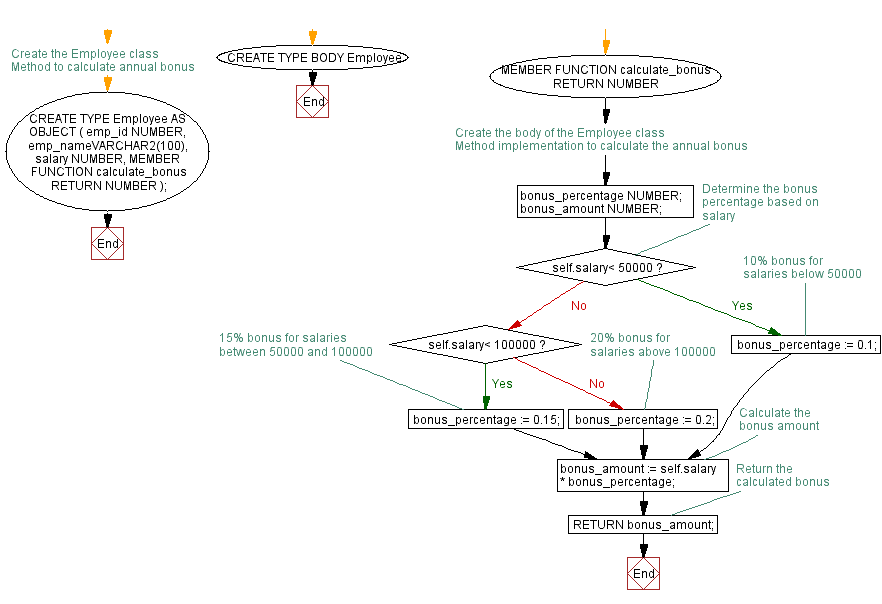
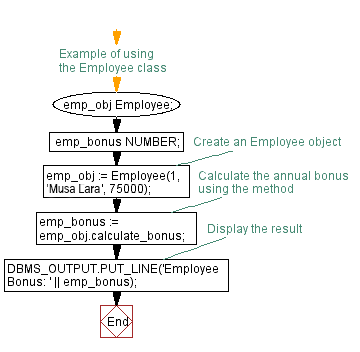
Explanation:
In this example, an Employee object with ID 1, name "Musa Lara," and a salary of 75000 is creates. Then by calling the calculate_bonus method on the Employee object it calculates and display the annual bonus.
Previous: Method to calculate annual bonus based on salary in PL/SQL's "Employee" Class.
Next: Creating a Subclass "Manager" in PL/SQL Inheriting from "Employee" Class.
What is the difficulty level of this exercise?