Methods in PL/SQL for a "Person" class with display and age update
PL/SQL OOP: Exercise-2 with Solution
Write a PL/SQL code to Implement methods in the "Person" class to display the details and update the age.
Sample Solution:
PL/SQL Code:
-- Define a custom data type "Person" using the OBJECT keyword.
CREATE OR REPLACE TYPE Person AS OBJECT (
id NUMBER, -- Attribute to store the unique identifier for the person.
name VARCHAR2(100), -- Attribute to store the name of the person (up to 100 characters).
age NUMBER, -- Attribute to store the age of the person.
-- Define a member function "displayDetails" to return details about the person.
MEMBER FUNCTION displayDetails RETURN VARCHAR2,
-- Define a member procedure "updateAge" to update the age of the person.
MEMBER PROCEDURE updateAge(newAge NUMBER)
);
/
The above code defines a PL/SQL object type Person with the required attributes with two methods (displayDetails and updateAge).
The displayDetails method returns a string containing the person's details and updateAge method allows updating the person's age.
Now, let's implement the body of the Person class:
PL/SQL Code:
-- Define the body of the custom data type "Person".
CREATE OR REPLACE TYPE BODY Person AS
-- Implement a member function "displayDetails" to return details about the person.
MEMBER FUNCTION displayDetails RETURN VARCHAR2 IS
BEGIN
RETURN 'Person ID: ' || id || ', Name: ' || name || ', Age: ' || age;
END;
-- Implement a member procedure "updateAge" to update the age of the person.
MEMBER PROCEDURE updateAge(newAge NUMBER) IS
BEGIN
age := newAge;
END;
END;
/
Here's an example of how to use the Person class:
PL/SQL Code:
-- Declare two instances of the "Person" object.
p1 Person := Person(1, 'Clay Frank', 30); -- Person with ID 1, name "Clay Frank", and age 30.
p2 Person := Person(2, 'Rory Peters', 25); -- Person with ID 2, name "Rory Peters", and age 25.
BEGIN
-- Display details of the first person.
DBMS_OUTPUT.PUT_LINE(p1.displayDetails());
-- Display details of the second person.
DBMS_OUTPUT.PUT_LINE(p2.displayDetails());
-- Update age of the first person.
p1.updateAge(31);
-- Display updated details of both persons.
DBMS_OUTPUT.PUT_LINE('After updating age:');
DBMS_OUTPUT.PUT_LINE(p1.displayDetails());
DBMS_OUTPUT.PUT_LINE(p2.displayDetails());
END;
/
Sample Output:
Statement processed. Person ID: 1, Name: Clay Frank, Age: 30 Person ID: 2, Name: Rory Peters, Age: 25 After updating age: Person ID: 1, Name: Clay Frank, Age: 31 Person ID: 2, Name: Rory Peters, Age: 25
Flowchart:
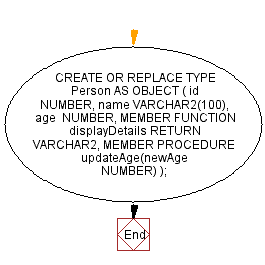
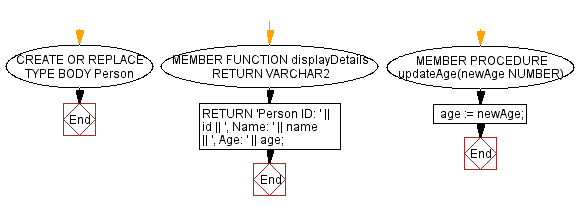
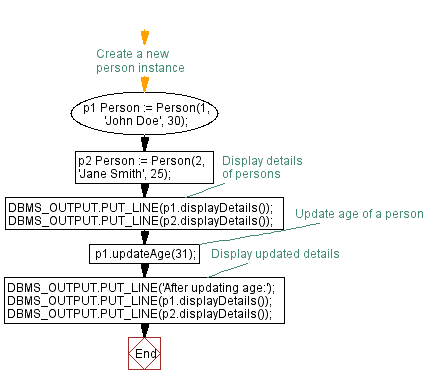
Explanation:
The said code in Oracle's PL/SQL that demonstrates the implementation of a simple "Person" class with methods to display person details and update their age.
The two instances of the Person class p1 and p2 are created to display their details, then update the age of p1 using the updateAge method, and finally display the updated details of both persons.
The DBMS_OUTPUT.PUT_LINE statements are used to print the output to the console. To see the output, you may need to enable the output display in your SQL client.
Previous: Creating a Person Class in PL/SQL - Object-Oriented Programming.
Next: Method to calculate annual bonus based on salary in PL/SQL's "Employee" Class.
What is the difficulty level of this exercise?
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/plsql-exercises/oop/plsql-oop-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics