Creating a Person Class in PL/SQL - Object-Oriented Programming
PL/SQL OOP: Exercise-1 with Solution
Write a PL/SQL code to create a class for a "Person" with attributes such as name, age, and address.
Sample Solution:
PL/SQL Code:
-- Create a custom data type named "Person" using the OBJECT keyword.
-- This data type will have three attributes: name, age, and address.
CREATE TYPE Person AS OBJECT (
name VARCHAR2(50), -- Attribute to store the name of the person (up to 50 characters).
age NUMBER, -- Attribute to store the age of the person.
address VARCHAR2(100) -- Attribute to store the address of the person (up to 100 characters).
);
/
The saidcode is not directly executable as standalone PL/SQL code. It is a user-defined object type called "Person.
Here's the example of how you can create a PL/SQL block to demonstrate the executable usage of the "Person" object type:
PL/SQL Code:
-- Create the "Person" object type
CREATE TYPE Person AS OBJECT (
name VARCHAR2(50), -- Attribute to store the name of the person (up to 50 characters).
age NUMBER, -- Attribute to store the age of the person.
address VARCHAR2(100) -- Attribute to store the address of the person (up to 100 characters).
);
/
-- Create a PL/SQL block to demonstrate usage
-- Declare a variable of type "Person"
DECLARE
p1 Person;
BEGIN
-- Instantiate the "Person" object
p1 := Person('John Doe', 30, '123 Main Street');
-- Display the attributes of the "Person" object
DBMS_OUTPUT.PUT_LINE('Name: ' || p1.name);
DBMS_OUTPUT.PUT_LINE('Age: ' || p1.age);
DBMS_OUTPUT.PUT_LINE('Address: ' || p1.address);
END;
/
Sample Output:
Type created. Statement processed. Name: John Doe Age: 30 Address: 123 Main Street
Flowchart:
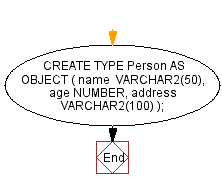
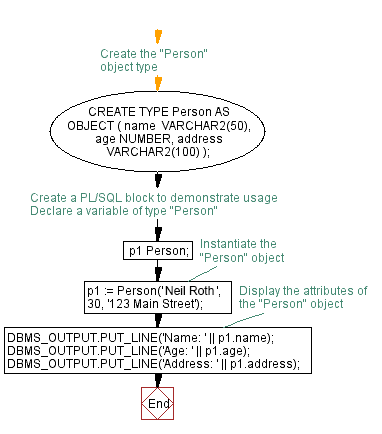
Explanation:
The said code in Oracle's PL/SQL that defined the structure of a "Person" class with its attributes. Now this "Person" object type can be used to create instances of the class, each representing a specific person with their name, age, and address.
This object type represents the blueprint for a "Person" class in the Object-Oriented Programming (OOP) paradigm.
Person is the name of our object type, which will serve as the class name for our "Person" class.
The attributes of the "Person" class are, the name is a VARCHAR2 attribute representing the person's name. It can hold up to 50 characters, the age is a NUMBER attribute representing the person's age and the address is a VARCHAR2 attribute representing the person's address. It can hold up to 100 characters.
Previous: PL/SQL OOP Exercises Home.
Next: Methods in PL/SQL for a "Person" class with display and age update.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics