PL/SQL Cursor Exercises: Display the last name, first name and overpaid amount by adding formal parameters
PL/SQL Cursor: Exercise-41 with Solution
Write a PL/SQL block to display the last name, first name and overpaid amount by adding formal parameters and specify a default values for the added parameters.
Sample Solution:
Table: employees
employee_id integer first_name varchar(25) last_name varchar(25) email archar(25) phone_number varchar(15) hire_date date job_id varchar(25) salary integer commission_pct decimal(5,2) manager_id integer department_id integer
PL/SQL Code:
Sample Output:
SQL> / --------------------------------- Extra Salary paid to Programmers: --------------------------------- Hunold, Alexander (by 3000) ----------------------------------- Extra Salary paid to Stock Manager: ----------------------------------- Mourgos, Kevin (by 800) PL/SQL procedure successfully completed.
Flowchart:
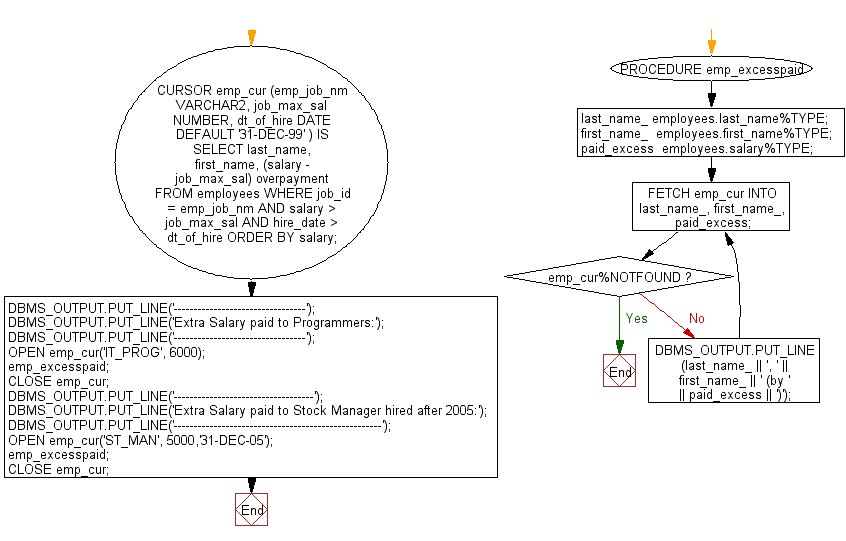
Improve this sample solution and post your code through Disqus
Previous: Write a PL/SQL block to find out the start date for current job of a specific employee.
Next: Write a block in PL/SQL to display the first name, job title and start date of employees.
What is the difficulty level of this exercise?
Based on 340 votes, average difficulty level of this exercise is Easy
.