PL/SQL Cursor Exercises: FETCH single record and single column from a table
PL/SQL Cursor: Exercise-15 with Solution
Write a program in PL/SQL to FETCH single record and single column from a table.
Sample Solution:
Table: employees
employee_id integer first_name varchar(25) last_name varchar(25) email archar(25) phone_number varchar(15) hire_date date job_id varchar(25) salary integer commission_pct decimal(5,2) manager_id integer department_id integer
PL/SQL Code:
DECLARE
v_emp_name VARCHAR2(50);
CURSOR emp_cur_name IS
SELECT first_name
FROM employees
WHERE employee_id = 105;
BEGIN
OPEN emp_cur_name;
FETCH emp_cur_name
INTO v_emp_name;
dbms_output.put_line('The name of the employee is:’
|| v_emp_name);
CLOSE emp_cur_name;
END;
/
Sample Output:
SQL> / The name of the employee is:David PL/SQL procedure successfully completed.
Flowchart:
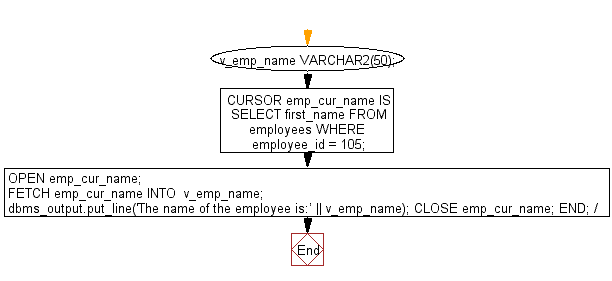
Improve this sample solution and post your code through Disqus
Previous: Create a PL/SQL block to increase salary of employees in the department 50 using WHERE CURRENT OF clause.
Next: Write a program in PL/SQL to FETCH more than one record and single column from a table.
What is the difficulty level of this exercise?