PL/SQL Control Statement Exercises: Display which day is a specific date
PL/SQL Control Statement: Exercise-15 with Solution
Write a PL/SQL program to display which day is a specific date
Sample Solution:
PL/SQL Code:
SET serveroutput ON
DECLARE
t_dt DATE := To_date('&input_a_date', 'DD-MON-YYYY');
t_day VARCHAR2(1);
BEGIN
t_day := To_char(t_dt, 'D');
CASE t_day
WHEN '1' THEN
dbms_output.Put_line ('The date you entered is Sunday.');
WHEN '2' THEN
dbms_output.Put_line ('The date you entered is Monday.');
WHEN '3' THEN
dbms_output.Put_line ('The date you entered is Tuesday.');
WHEN '4' THEN
dbms_output.Put_line ('The date you entered is Wednesday.');
WHEN '5' THEN
dbms_output.Put_line ('The date you entered is Thursday.');
WHEN '6' THEN
dbms_output.Put_line ('The date you entered is Friday.');
WHEN '7' THEN
dbms_output.Put_line ('The date you entered is Saturday.');
END CASE;
END;
/
Sample Output:
SQL> / SQL> / Enter value for input_a_date: 15-may-2018 old 2: t_dt DATE := To_date('&input_a_date', 'DD-MON-YYYY'); new 2: t_dt DATE := To_date('15-may-2018', 'DD-MON-YYYY'); The date you entered is Tuesday. PL/SQL procedure successfully completed.
Sample Output:
Enter value for input_a_date: 14-sep-2017 old 2: t_dt DATE := To_date('&input_a_date', 'DD-MON-YYYY'); new 2: t_dt DATE := To_date('14-sep-2017', 'DD-MON-YYYY'); The date you entered is Thursday. PL/SQL procedure successfully completed.
Flowchart:
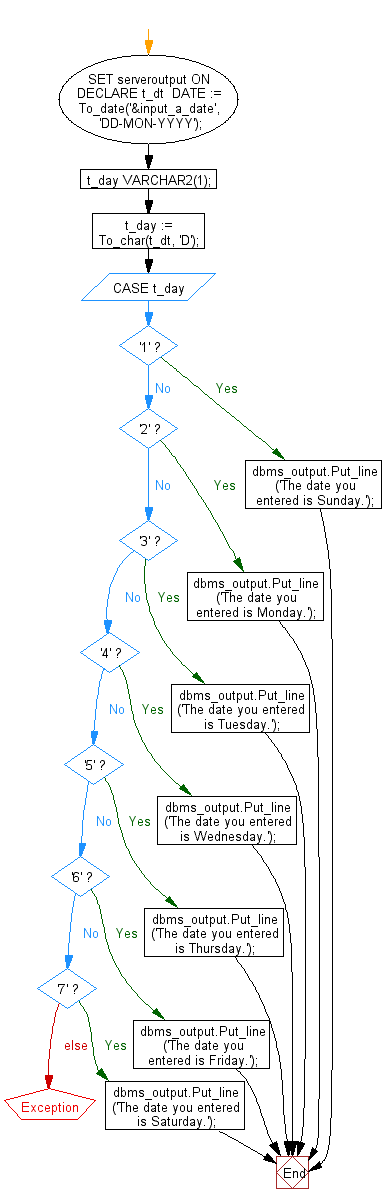
Improve this sample solution and post your code through Disqus
Previous: Write a PL/SQL program to convert a temperature in scale Fahrenheit to Celsius and vice versa.
Next: Write a program in PL/SQL to print the value of a variable inside and outside a loop using LOOP EXIT statement.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics