PHP Searching and Sorting Algorithm: Cocktail sort
Write a PHP program to sort a list of elements using Cocktail sort.
Cocktail shaker sort (also known as bidirectional bubble sort, cocktail sort, shaker sort, ripple sort, shuffle sort, or shuttle sort ) is a variation of bubble sort that is both a stable sorting algorithm and a comparison sort. The algorithm differs from a bubble sort in that it sorts in both directions on each pass through the list. This sorting algorithm is only marginally more difficult to implement than a bubble sort and solves the problem of turtles in bubble sorts. It provides only marginal performance improvements, and does not improve asymptotic performance; like the bubble sort, it is not of practical interest, though it finds some use in education.
Visualization of shaker sort :
Sample Solution :
PHP Code :
<?php
// Function to perform Cocktail Shaker Sort on an array
function cocktailSort($my_array)
{
// If the input is a string, convert it to an array of characters
if (is_string($my_array))
$my_array = str_split(preg_replace('/\s+/','',$my_array));
// Loop until no swaps are made
do {
$swapped = false; // Flag to track if any elements were swapped
// Forward pass through the array
for($i = 0; $i < count($my_array); $i++) {
// Check if the next element exists
if(isset($my_array[$i+1])) {
// Compare adjacent elements and swap if necessary
if($my_array[$i] > $my_array[$i+1]) {
list($my_array[$i], $my_array[$i+1]) = array($my_array[$i+1], $my_array[$i]);
$swapped = true; // Set swapped flag to true
}
}
}
// If no swaps were made in the forward pass, break out of the loop
if ($swapped == false) break;
$swapped = false; // Reset swapped flag
// Backward pass through the array
for($i = count($my_array) - 1; $i >= 0; $i--) {
// Check if the previous element exists
if(isset($my_array[$i-1])) {
// Compare adjacent elements and swap if necessary
if($my_array[$i] < $my_array[$i-1]) {
list($my_array[$i], $my_array[$i-1]) = array($my_array[$i-1], $my_array[$i]);
$swapped = true; // Set swapped flag to true
}
}
}
} while($swapped); // Continue loop until no swaps are made
return $my_array; // Return the sorted array
}
// Test array
$test_array = array(3, 0, 2, 5, -1, 4, 1);
echo "Original Array :\n";
echo implode(', ',$test_array ); // Display original array
echo "\nSorted Array\n:";
echo implode(', ',cocktailSort($test_array)). PHP_EOL; // Display sorted array
?>
Output:
3, 0, 2, 5, -1, 4, 1 Sorted Array : -1, 0, 1, 2, 3, 4, 5
Explanation:
In the exercise above,
- The code defines a function "cocktailSort()" that implements the Cocktail Shaker Sort algorithm.
- The function takes an array '$my_array' as input and sorts it in ascending order.
- If the input is a string, it converts it to an array of characters.
- The function uses a do-while loop to perform the sorting.
- Inside the loop, it performs two passes through the array: a forward pass and a backward pass.
- In each pass, it compares adjacent elements and swaps them if they are in the wrong order.
- The loop continues until no more swaps are made, indicating the array is sorted.
- Finally, the sorted array is returned.
- The code then tests the sorting function with a sample array, displaying the original and sorted arrays.
Flowchart :
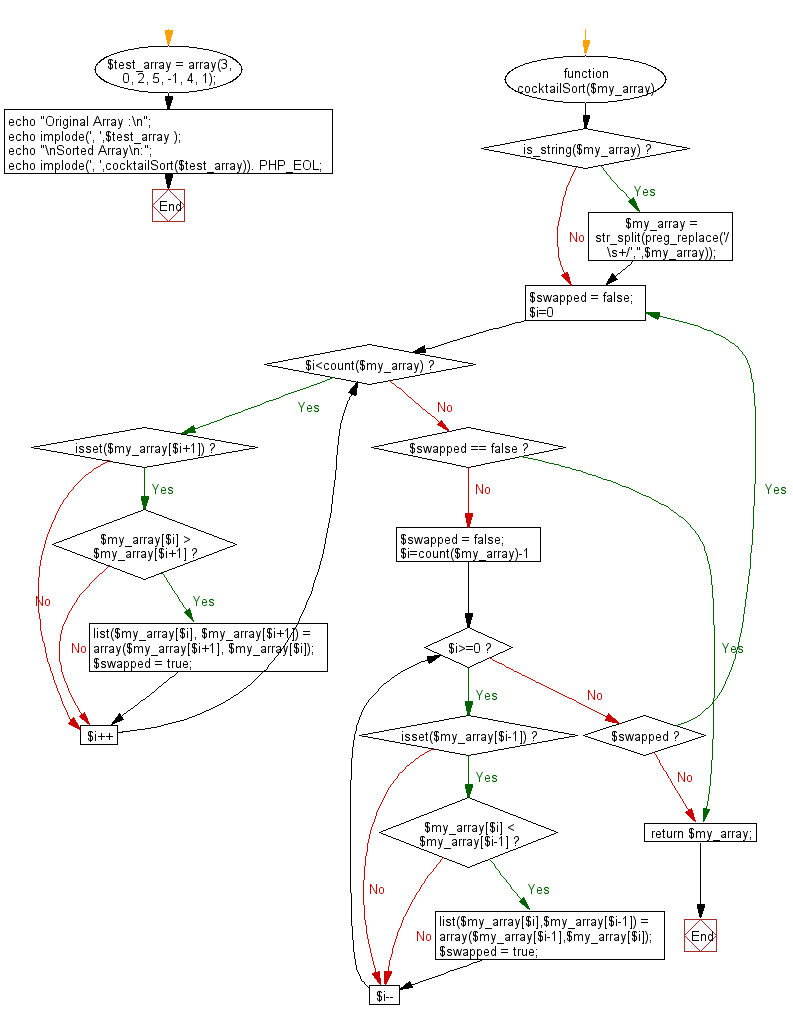
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to sort a list of elements using Bubble sort.
Next: Write a PHP program to sort a list of elements using Comb sort.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics