PHP Searching and Sorting Algorithm: Selection sort
PHP Searching and Sorting Algorithm: Exercise-4 with Solution
Write a PHP program to sort a list of elements using Selection sort.
The selection sort improves on the bubble sort by making only one exchange for every pass through the list.
Visual Presentation : Selection Sort
Sample Solution :
PHP Code :
<?php
// Function to perform selection sort on an array
function selection_sort($data)
{
// Loop through the array
for ($i = 0; $i < count($data) - 1; $i++) {
$min = $i;
// Find the minimum element in the remaining unsorted array
for ($j = $i + 1; $j < count($data); $j++) {
if ($data[$j] < $data[$min]) {
$min = $j;
}
}
// Swap the minimum element with the first unsorted element
$data = swap_positions($data, $i, $min);
}
return $data;
}
// Function to swap two elements in an array
function swap_positions($data1, $left, $right)
{
// Backup the value of the element at the right position
$backup_old_data_right_value = $data1[$right];
// Swap the elements at the left and right positions
$data1[$right] = $data1[$left];
$data1[$left] = $backup_old_data_right_value;
return $data1;
}
// Input array to be sorted
$my_array = array(3, 0, 2, 5, -1, 4, 1);
// Print the original array
echo "Original Array :\n";
echo implode(', ', $my_array);
echo "\nSorted Array :\n";
// Perform selection sort on the array and print the sorted array
echo implode(', ', selection_sort($my_array)) . PHP_EOL;
?>
Output:
Original Array : 3, 0, 2, 5, -1, 4, 1 Sorted Array : -1, 0, 1, 2, 3, 4, 5
Explanation:
In the exercise above,
- Function selection_sort($data):
- This function takes an array '$data' as input and sorts it using the selection sort algorithm.
- It iterates through the array and selects the minimum element in each iteration, swapping it for the element at the current position.
- Function swap_positions($data1, $left, $right):
- This function takes an array '$data1' and two indices '$left' and '$right' as input.
- It swaps the elements at positions '$left' and '$right' in the array '$data1'.
- Main code:
- An input array '$my_array' is defined with unsorted numbers.
- The original array is printed.
- The "selection_sort()" function is called to sort the array.
- The sorted array is printed.
- Selection Sorting Algorithm:
- The selection sort algorithm works by dividing the input array into two subarrays: sorted and unsorted.
- In each iteration, it finds the minimum element from the unsorted subarray and places it at the beginning of the sorted subarray.
- This process continues until the entire array is sorted.
- Code execution:
- The "selection_sort()" function iterates through the array.
- In each iteration, it finds the minimum element in the unsorted part of the array using nested loops.
- It swaps the minimum element with the current element at the beginning of the unsorted part.
- After iterations, the array is sorted, and the sorted array is returned.
Flowchart :
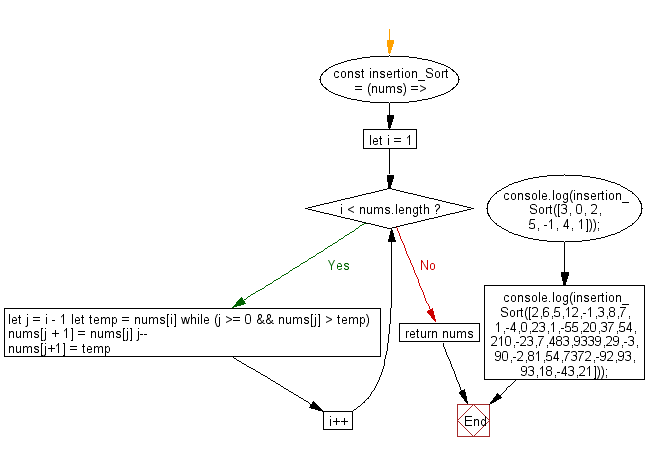
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous:Write a PHP program to sort a list of elements using Insertion sort.
Next: Write a PHP program to sort a list of elements using Shell sort.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics