PHP String Exercises : Format values in currency style
Write a PHP script to format values in currency style.
Sample values: value1 = 65.45, value2 = 104.35
Sample Solution:
PHP Code:
<?php
$value1 = 65.45; // Assigns the value 65.45 to the variable $value1
$value2 = 104.35; // Assigns the value 104.35 to the variable $value2
// Uses sprintf() function to format the sum of $value1 and $value2 with two decimal places
// %1.2f specifies the format: 1 total width, 2 decimal places
echo sprintf("%1.2f", $value1+$value2)."\n"; // Echoes the formatted sum to the output followed by a newline.
?>
Output:
169.80
Explanation:
In the exercise above,
- Two variables, '$value1' and '$value2', are initialized with floating-point numbers 65.45 and 104.35 respectively. These variables represent numerical values.
- The "sprintf()" function formats the sum of '$value1' and '$value2'. Inside the "sprintf()" function:
- %1.2f is a format specifier where:
- %f is a placeholder for a floating-point number.
- 1.2 specifies the format: 1 total width (including the decimal point and digits before and after it), and 2 decimal places after the decimal point.
- $value1 + $value2 calculates the sum of the two values.
- The formatted result is then echoed to output, followed by a newline character (\n).
Flowchart :
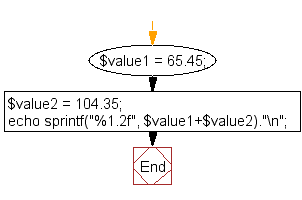
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP script to get the last three characters of a string.
Next: Write a PHP script to generate simple random password [do not use rand() function] from a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics