PHP String Exercises: Select first 5 words from the specified string
PHP String: Exercise-24 with Solution
Write a PHP script to select first 5 words from the following string.
Sample String : 'The quick brown fox jumps over the lazy dog'
Visual Presentation:
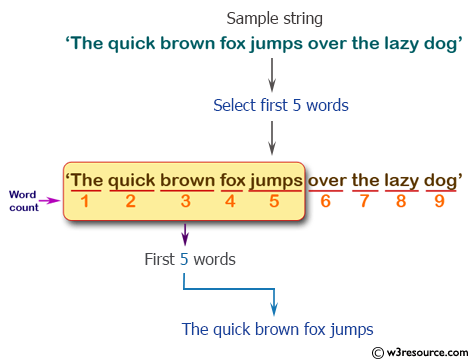
Sample Solution:
PHP Code:
<?php
$my_string = 'The quick brown fox jumps over the lazy dog';
// Define the original string.
echo implode(' ', array_slice(explode(' ', $my_string), 0, 5))."\n";
// Split the string into an array of words, take the first 5 elements, join them with a space, and output the result.
?>
Sample Output:
The quick brown fox jumps
Explanation:
In the exercise above,
- explode(' ', $my_string): Splits the string '$my_string' into an array of words using space ' ' as the delimiter.
- array_slice(..., 0, 5): Retrieves the first 5 elements from the array obtained from the explode() function.
- implode(' ', ...): Joins the selected elements from the array into a string with space ' ' as the glue.
- Finally, it echoes out the modified string, which consists of the first 5 words of the original string separated by spaces.
Flowchart :
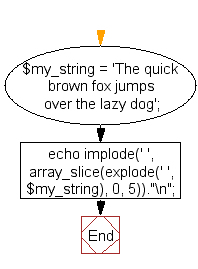
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP script to replace multiple characters from the specified string.
Next: Write a PHP script to remove comma(s) from the specified numeric string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics