PHP String Exercises: Find the first character that is different between two strings
Write a PHP script to find the first character that is different between two strings.
String1 : 'football'
String2 : 'footboll'
Visual Presentation:
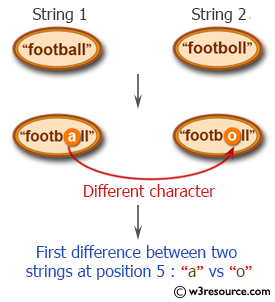
Sample Solution:
PHP Code:
<?php
// Define two strings to compare
$str1 = 'football';
$str2 = 'footboll';
// Calculate the position of the first difference between the two strings
$str_pos = strspn($str1 ^ $str2, "\0");
// Output the position of the first difference along with the characters at that position
printf('First difference between two strings at position %d: "%s" vs "%s"',
$str_pos, $str1[$str_pos], $str2[$str_pos]);
printf("\n");
?>
Output:
First difference between two strings at position 5: "a" vs "o"
Explanation:
In the exercise above,
- String comparison:
- Two strings are defined: $str1 with the value 'football' and $str2 with the value 'footboll'.
- First difference calculation:
- The strspn() function calculates the position of the first difference between the two strings.
- The bitwise XOR operator () compares each character of the strings.
- The strspn() function returns the length of the initial segment of $str1 ^ $str2 consisting of characters not present in the mask. This is "\0" (the null character).
- Output:
- The position of the first difference is printed along with the characters at that position in both strings.
Flowchart :
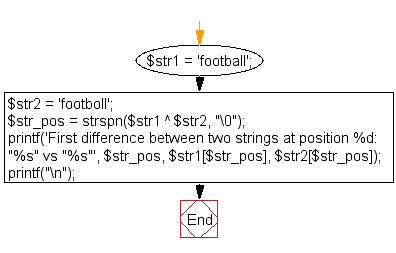
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP script to replace the first 'the' of the following string with 'That'.
Next: Write a PHP script to put a string in an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.