PHP Regular Expression Exercises: Extract text (within parenthesis) from a string
6. Extract text (within parenthesis) from a string
Write a PHP script to extract text (within parenthesis) from a string.
Sample strings : 'The quick brown [fox].'
Visual Presentation:
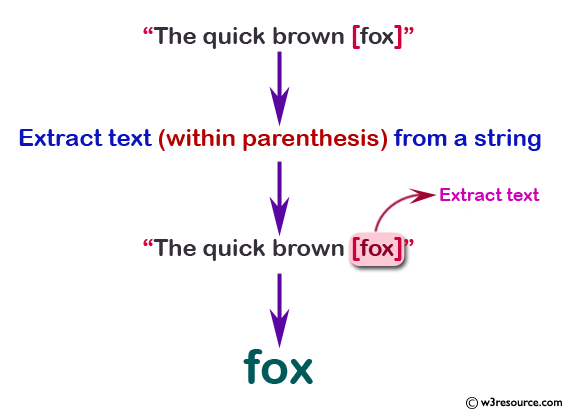
Sample Solution:
PHP Code:
<?php
// Define the input text
$my_text = 'The quick brown [fox].';
// Use preg_match to find the substring enclosed in square brackets
preg_match('#\[(.*?)\]#', $my_text, $match);
// Print the matched substring captured within the square brackets
print $match[1]."\n";
?>
Output:
fox
Explanation:
In the exercise above,
- <?php: PHP opening tag.
- $my_text = 'The quick brown [fox].';: Assigns a string containing square brackets to the variable $my_text.
- preg_match('#\[(.*?)\]#', $my_text, $match);: Uses a regular expression pattern to match text enclosed within square brackets. The "preg_match()" function searches for this pattern in '$my_text' and stores the result in the '$match' array.
- print $match[1]."\n";: Prints the matched substring captured within the square brackets.
Flowchart :
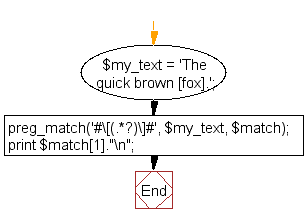
For more Practice: Solve these Related Problems:
- Write a PHP script to extract text from multiple sets of parentheses within a string and return them as an array.
- Write a PHP function that extracts only the outermost set of parenthesis content, ignoring any nested ones.
- Write a PHP function to extract and count words within parentheses, ignoring other parts of the string.
- Write a PHP program to extract all unique substrings enclosed within parentheses from a complex multiline string.
Go to:
PREV : Remove new lines (characters) from a string.
NEXT : Remove all characters from a string except a-z A-Z 0-9 or " ".
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.