PHP Math Exercises: Convert Arabic numbers to Roman numerals
Write a PHP function to convert Arabic numbers to Roman numerals.
Visual Presentation:
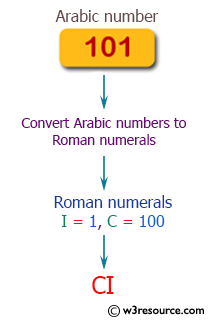
Sample Solution:
PHP Code:
<?php
function arabic_rome($num)
{
$c = 'IVXLCDM'; // Roman numeral characters
for ($x = 5, $y = $result = ''; $num; $y++, $x ^= 7) { // Loop until $num is not zero
$o = $num % $x; // Remainder of $num divided by $x
$num = $num / $x ^ 0; // Integer division of $num by $x
// Loop to append the corresponding Roman numeral characters to $result
for (; $o--; $result = $c[$o > 2 ? $y + $num - ($num = -2) + $o = 1 : $y] . $result);
}
return $result; // Return the Roman numeral representation of the input number
}
print_r(arabic_rome(101) . "\n"); // Test the function with input 101
?>
Output:
CI
Explanation:
In the exercise above,
- function arabic_rome($num) {: This line defines a function named "arabic_rome()" that takes an Arabic numeral '$num' as input.
- $c = 'IVXLCDM';: This line defines a string '$c' containing Roman numeral characters from 1 to 1000 (I, V, X, L, C, D, M).
- for ($x = 5, $y = $result = ''; $num; $y++, $x ^= 7) {: This line initializes variables '$x', '$y', and '$result'. It then enters a loop that continues until '$num' becomes zero. During each iteration, '$y' is incremented and '$x' is bitwise XORed with 7 (alternating between 2 and 5).
- $o = $num % $x;: This line calculates the remainder of '$num' divided by '$x' and stores it in '$o'.
- $num = $num / $x ^ 0;: This line performs integer division of '$num' by '$x' and updates '$num' with the quotient.
- The subsequent "for" loop iterates '$o' times and appends the corresponding Roman numeral characters to '$result' based on the value of '$o' and '$y'.
- return $result;: This line returns the final Roman numeral representation of the input number.
- The "print_r" statement tests the "arabic_rome()" function with the input number 101 and prints the result.
Flowchart :
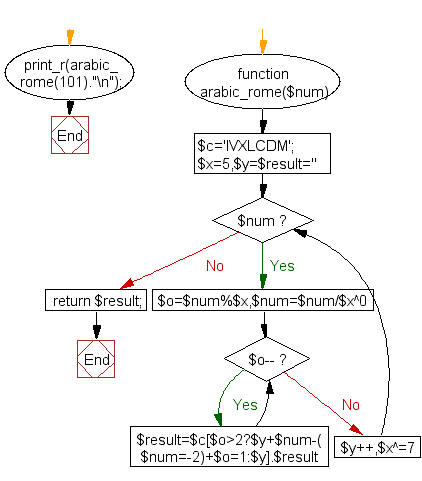
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP function to round a float away from zero to a specified number of decimal places.
Next: Write a PHP function to get random float numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics