PHP Math Exercises: Round a float away from zero to a specified number of decimal places
8. Round Float Away from Zero
Write a PHP function to round a float away from zero to a specified number of decimal places.
Sample Data :
(78.78001, 2)
(8.131001, 2)
(0.586001, 4)
(-.125481, 3)
-.125481
Sample Solution:
PHP Code:
<?php
function roundout($value, $places = 0) {
if ($places < 0) {
$places = 0; // Ensure places is non-negative
}
$x = pow(10, $places); // Calculate the multiplier
// Round the value based on its sign and the multiplier, then divide by the multiplier
return ($value >= 0 ? ceil($value * $x) : floor($value * $x)) / $x;
}
// Test the roundout function with different values and precision
echo roundout(78.78001, 2) . "\n";
echo roundout(8.131001, 2) . "\n";
echo roundout(0.586001, 4) . "\n";
echo roundout(-0.125481, 3) . "\n";
echo roundout(-0.125481);
?>
Output:
78.79 8.14 0.5861 -0.126 -1
Explanation:
In the exercise above,
- 'function roundout($value, $places = 0) {': This line defines a function named 'roundout' that takes two parameters: '$value' (the number to be rounded) and '$places' (the number of decimal places to round to, defaulting to 0 if not provided).
- 'if ($places < 0) { $places = 0; }': This line ensures that the number of decimal places is non-negative. If '$places' is less than 0, it is set to 0.
- '$x = pow(10, $places);': This line calculates the multiplier '$x' by raising 10 to the power of the number of decimal places.
- 'return ($value >= 0 ? ceil($value $x) : floor($value $x)) / $x;': This line rounds the value based on its sign and the multiplier '$x'. If '$value' is non-negative, it is rounded up using 'ceil()'; otherwise, it is rounded down using 'floor()'. The result is then divided by the multiplier to obtain the rounded value.
- The subsequent 'echo' statements test the 'roundout' function with different input values and precision levels, and display the rounded results.
Flowchart :
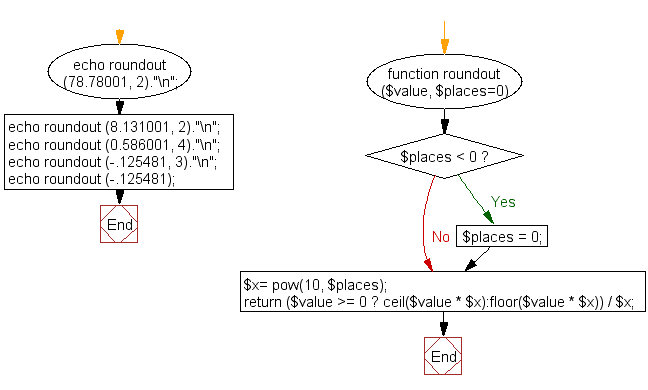
For more Practice: Solve these Related Problems:
- Write a PHP function to round a floating-point number away from zero to a specified precision without using built-in rounding functions.
- Write a PHP script to process an array of floats, rounding each away from zero and returning the results in an associative array with original indexes.
- Write a PHP program to compare the outputs of a custom "round away from zero" function with PHP's round() using different precision parameters.
- Write a PHP function that rounds a float away from zero by first checking its sign and then applying a floor/ceil operation accordingly.
Go to:
PREV : Find Earliest and Latest Dates.
NEXT : Arabic to Roman Numerals Conversion.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.