PHP Math Exercises: Rounds the specified values with 1 decimal digit precision
PHP math: Exercise-2 with Solution
Write a PHP script which rounds the following values with 1 decimal digit precision.
Sample values :
1.65
1.65
-1.54
Visual Presentation:
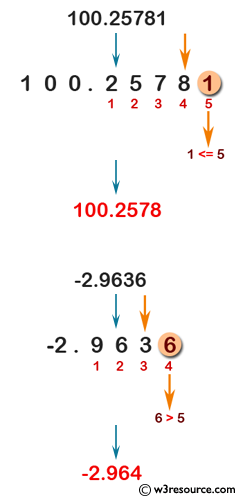
Sample Solution:
PHP Code:
<?php
echo round( 1.65, 1, PHP_ROUND_HALF_UP)."\n"; // Rounds up to nearest even number: 1.7
echo round( 1.65, 1, PHP_ROUND_HALF_DOWN)."\n"; // Rounds down to nearest even number: 1.6
echo round(-1.54, 1, PHP_ROUND_HALF_EVEN)."\n"; // Rounds to nearest even number: -1.5
?>
Output:
1.7 1.6 -1.5
Explanation:
In the exercise above,
- echo round( 1.65, 1, PHP_ROUND_HALF_UP)."\n";: This line rounds the number 1.65 to 1 decimal place using the 'PHP_ROUND_HALF_UP' rounding mode, which means rounding away from zero. So, 1.65 rounds up to 1.7.
- echo round( 1.65, 1, PHP_ROUND_HALF_DOWN)."\n";: This line rounds the number 1.65 to 1 decimal place using the 'PHP_ROUND_HALF_DOWN' rounding mode, which means rounding towards zero. So, 1.65 rounds down to 1.6.
- echo round(-1.54, 1, PHP_ROUND_HALF_EVEN)."\n";: This line rounds the number -1.54 to 1 decimal place using the 'PHP_ROUND_HALF_EVEN' rounding mode, which means rounding to the nearest even number. So, -1.54 rounds to -1.5.
Flowchart :
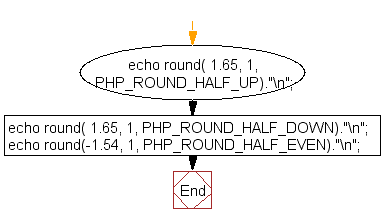
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP script to find the maximum and minimum marks from the specified set of arrays.
Next: Write a PHP script to generate random 11 characters string of letters and numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics