PHP Math Exercises: Create a human-readable random string for a captcha
PHP math: Exercise-11 with Solution
Write a PHP function to create a human-readable random string for a captcha.
Sample Solution:
PHP Code:
<?php
// Define a function named random_string with a default length of 5
function random_string($length = 5)
{
// Define a string of characters without vowels and 'x'
$chars = 'bcdfghjklmnpqrstvwxyz';
// Initialize an empty string to store the result
$result = '';
// Iterate through the specified length to generate random characters
for ($x = 0; $x < $length; $x++)
{
// Alternate between consonants and vowels while generating characters
$result .= ($x % 2) ? $chars[mt_rand(13, 21)] : $chars[mt_rand(0, 12)];
}
// Return the generated random string
return $result;
}
// Call the random_string function and echo the result
echo random_string();
?>
Output:
rasyn
Explanation:
In the exercise above,
- function random_string($length = 5): This line defines a function named "random_string()" with one parameter '$length', which has a default value of 5 if not provided.
- $chars = 'bcdfghjklmnpqrstvwxyz';: This line defines a string of characters containing consonants excluding 'x'. Vowels and 'x' are omitted to avoid inappropriate strings.
- $result = '';: This line initializes an empty string to store the generated random string.
- for ($x = 0; $x < $length; $x++): This line starts a loop that iterates from 0 to the specified length, generating random characters.
- $result .= ($x % 2) ? $chars[mt_rand(13, 21)] : $chars[mt_rand(0, 12)];: This line generates random characters alternately from consonants and vowels. It uses the modulus operator to determine whether the index of the character to be chosen is even or odd.
- return $result;: This line returns the generated random string.
- echo random_string();: This line calls the "random_string()" function without providing a length, so it uses the default length of 5, and echoes the generated random string.
Flowchart :
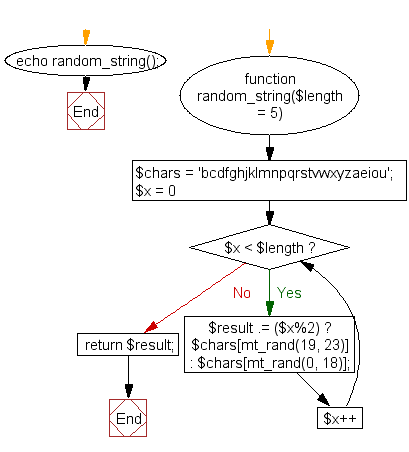
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP function to get random float numbers.
Next: Write a PHP function to get the distance between two points on the earth.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics