PHP function Exercises: Check whether a number is prime or not
PHP function: Exercise-2 with Solution
Write a PHP function to check whether a number is prime or not.
Note: A prime number (or a prime) is a natural number greater than 1 that has no positive divisors other than 1 and itself.
Visual Presentation:
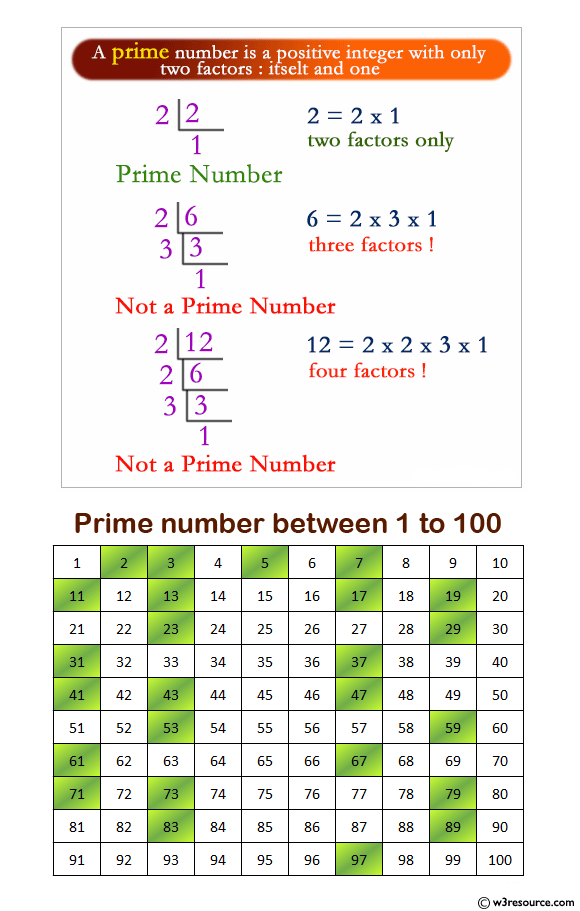
Sample Solution:
PHP Code:
<?php
// Function to check if a number is prime
function IsPrime($n)
{
// Loop through all numbers from 2 to n-1
for ($x = 2; $x < $n; $x++)
{
// If n is divisible by any number other than 1 and itself,
// it's not prime, so return 0
if ($n % $x == 0)
{
return 0;
}
}
// If no divisor found, n is prime, so return 1
return 1;
}
// Call the IsPrime function to check if 3 is prime
$a = IsPrime(3);
// Check the return value and print the result
if ($a == 0)
echo 'This is not a Prime Number.....' . "\n";
else
echo 'This is a Prime Number..' . "\n";
?>
Output:
This is a Prime Number..
Explanation:
In the exercise above,
- Define a function named "IsPrime()" which takes an integer parameter '$n'.
- Inside the function, there's a loop that iterates from 2 to one less than '$n'. This loop checks for divisors of '$n'.
- If '$n' is divisible by any number other than 1 and itself, the function returns 0, indicating that the number is not prime.
- If no divisor is found in the loop, the function returns 1, indicating that the number is prime.
- Outside the function, the "IsPrime()" function is called with an argument of 3, and the result is stored in the variable '$a'.
- The code then checks the value of '$a' and prints whether 3 is a prime number or not.
Flowchart :
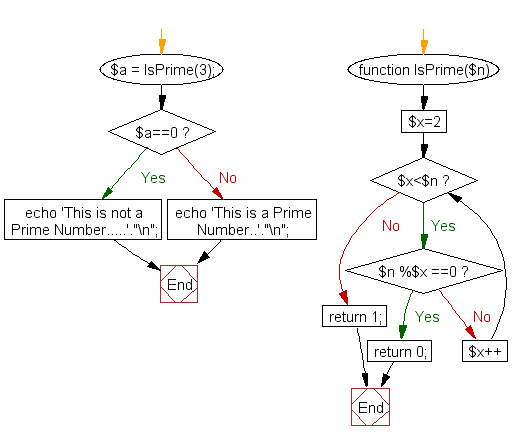
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a function to calculate the factorial of a number (a non-negative integer). The function accepts the number as an argument.
Next: Write a function to reverse a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/php-exercises/php-function-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics