PHP for loop Exercises: Print alphabet pattern R
30. Alphabet Pattern 'R'
Write a PHP program to print alphabet pattern 'R'.
Visual Presentation:
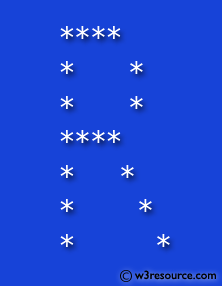
Sample Solution:
PHP Code:
<?php
// Loop for rows
for ($row=0; $row<7; $row++)
{
// Loop for columns
for ($column=0; $column<=7; $column++)
{
// Condition to determine whether to print '*' or ' '
if ($column == 1 or (($row == 0 or $row == 3) and $column > 1 and $column < 5) or ($column == 5 and $row != 0 and $row < 3) or ($column == $row - 1 and $row > 2))
echo "*"; // Print '*' if condition is met
else
echo " "; // Print ' ' if condition is not met
}
echo "\n"; // Move to the next line after each row is printed
}
?>
Output:
**** * * * * **** * * * * * *
Explanation:
In the exercise above,
- The code starts with a PHP opening tag <?php.
- It utilizes a nested loop structure to iterate over rows and columns to create a specific pattern.
- The outer loop (for ($row=0; $row<7; $row++)) controls the rows of the pattern, iterating from 0 to 6.
- Inside the outer loop, there's another loop (for ($column=0; $column<=7; $column++)) that controls the columns, iterating from 0 to 7.
- Within the inner loop, there's a conditional statement that determines whether to print an asterisk ('*') or a space ( ) based on the position of the current row and column indices.
- The condition checks multiple cases:
- If the column is at index 1.
- If the row is at index 0 or 3 and the column is between indices 1 and 4.
- If the column is at index 5 and the row is not at index 0 and less than 3.
- If the column index is equal to the row index minus 1 and the row index is greater than 2.
- If any of these conditions are met, an asterisk ('*') is echoed. Otherwise, a space ( ) is echoed.
- After printing each row, the code moves to the next line by echoing a newline character ('\n').
- Once all rows and columns are printed according to the pattern, the PHP code ends with a closing PHP tag ?>.
Flowchart :
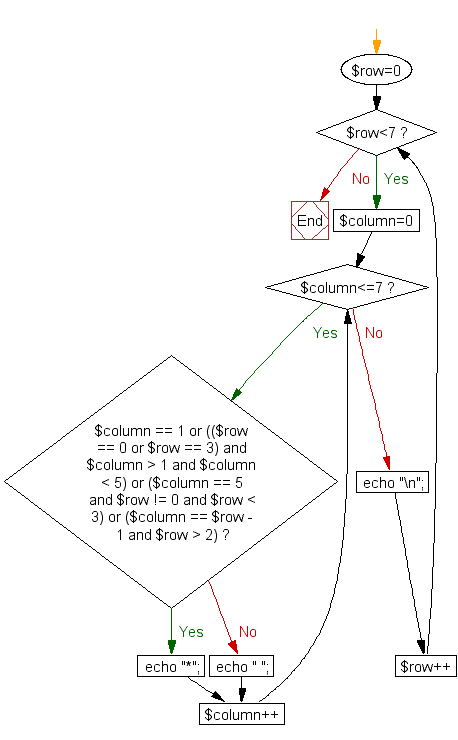
For more Practice: Solve these Related Problems:
- Write a PHP script to print the letter 'R' using loops, ensuring the diagonal leg is properly slanted.
- Write a PHP function to generate a scalable 'R' pattern that maintains proportion between the curved and diagonal parts.
- Write a PHP program to output an 'R' pattern that includes a bold vertical line and an angled leg using nested loops.
- Write a PHP script to construct a letter 'R' with adjustable spacing for the diagonal leg based on user input.
Go to:
PREV : Alphabet Pattern 'Q'.
NEXT : Alphabet Pattern 'S'.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.