PHP for loop Exercises: Construct a specific pattern, using nested for loop
Create a script to construct the following pattern, using nested for loop.
* * * * * * * * * * * * * * *
Visual Presentation:
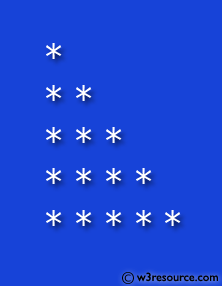
Sample Solution:
PHP Code:
<?php
// Outer loop to control the rows
for($x=1;$x<=5;$x++)
{
// Inner loop to control the columns within each row
for ($y=1;$y<=$x;$y++)
{
// Print a star for each column
echo "*";
// Add a space after each star, except for the last one in the row
if($y < $x)
{
echo " ";
}
}
// Move to the next line after printing each row
echo "\n";
}
?>
Output:
* * * * * * * * * * * * * * *
Explanation:
In the exercise above,
- The code starts with a PHP opening tag <?php.
- It contains an outer "for" loop that iterates through values from 1 to 5, controlling the rows of the pattern (for($x=1;$x<=5;$x++)).
- Inside the outer loop, there's an inner "for" loop that iterates through values from 1 to the current value of '$x', controlling the columns within each row (for ($y=1;$y<=$x;$y++)).
- Within the inner loop, a star ('*') is printed for each column (echo "";).
- Additionally, a space is printed after each star, except for the last one in the row, to create the triangular pattern (if($y < $x) { echo " "; }).
- After printing all the stars and spaces for a row, a newline character \n is printed to move to the next row (echo "\n";).
- This process continues until all rows have been printed.
- Finally, the PHP code ends with a closing tag ?>.
Flowchart :
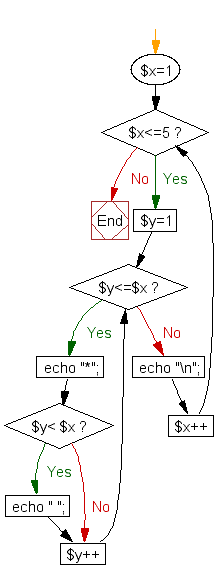
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Create a script using a for loop to add all the integers between 0 and 30 and display the sum.
Next: Create a script to construct the specific pattern, using a nested for loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics