PHP Date Exercises : Calculate the difference between two dates (in years, months, days)
Write a PHP script to calculate the difference between two dates.
Sample dates : 1981-11-04, 2013-09-04
Sample Solution:
PHP Code:
<?php
// Start date
$sdate = "1981-11-04";
// End date
$edate = "2013-09-04";
// Calculate the difference between the end date and the start date in seconds
$date_diff = abs(strtotime($edate) - strtotime($sdate));
// Calculate the number of years in the difference
$years = floor($date_diff / (365*60*60*24));
// Calculate the number of months in the remaining difference
$months = floor(($date_diff - $years * 365*60*60*24) / (30*60*60*24));
// Calculate the number of days in the remaining difference
$days = floor(($date_diff - $years * 365*60*60*24 - $months*30*60*60*24)/ (60*60*24));
// Output the calculated duration
printf("%d years, %d months, %d days", $years, $months, $days);
// Print a newline character for formatting
printf("\n");
?>
Output:
31 years, 10 months, 12 days
Explanation:
In the exercise above,
- Initialization: Two variables '$sdate' and '$edate' are initialized with start and end dates, respectively.
- Date Difference Calculation: The difference in seconds between the end date and the start date is calculated using "strtotime()" function.
- Conversion to Years, Months, and Days: The difference in seconds is converted into years, months, and days. The number of years is calculated by dividing the total seconds by the number of seconds in a year. Similarly, months and days are calculated from the remaining seconds after subtracting years and months.
- Output: The calculated duration (years, months, and days) is printed using "printf()" function with appropriate formatting.
Flowchart :
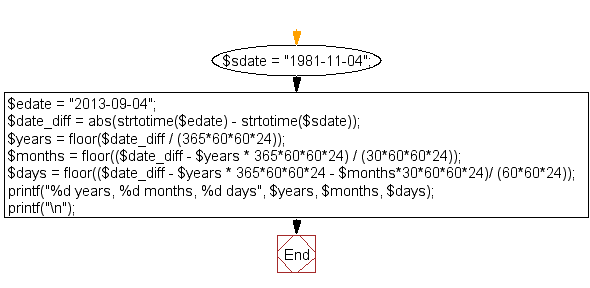
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP script to print the current date in the following format. To get current date's information you can use the date() function.
Next: Write a PHP script to convert a date from yyyy-mm-dd to dd-mm-yyyy.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.