PHP Date Exercises : Calculate the current age of a person
Write a PHP script to calculate the current age of a person.
Sample date of birth : 11.4.1987
Sample Solution:
PHP Code:
<?php
$bday = new DateTime('11.4.1987'); // Creating a DateTime object representing your date of birth.
$today = new Datetime(date('m.d.y')); // Creating a DateTime object representing today's date.
$diff = $today->diff($bday); // Calculating the difference between your date of birth and today's date.
printf(' Your age : %d years, %d month, %d days', $diff->y, $diff->m, $diff->d); // Displaying your age in years, months, and days.
printf("\n"); // Adding a new line for formatting.
?>
Output:
Your age : 30 years, 3 month, 0 days
N.B.: The result may varry for your system date and time.
Explanation:
In the exercise above,
- $bday = new DateTime('11.4.1987');: Creates a "DateTime" object representing your date of birth. The date is provided in the format 'month.day.year'.
- $today = new Datetime(date('m.d.y'));: Create a "DateTime" object representing today's date using the date function to get the current date in the format 'month.day.year'.
- $diff = $today->diff($bday);: Calculates the difference between your date of birth and today's date using the "diff()" method of the "DateTime" object. This returns a DateInterval object representing the difference.
- printf(' Your age : %d years, %d month, %d days', $diff->y, $diff->m, $diff->d);: Using "printf()" to format and display your age. '%d' is a placeholder for integers, and we pass the years, months, and days from the "DateInterval" object as arguments.
- printf("\n");: Adding a newline character for formatting.
Flowchart :
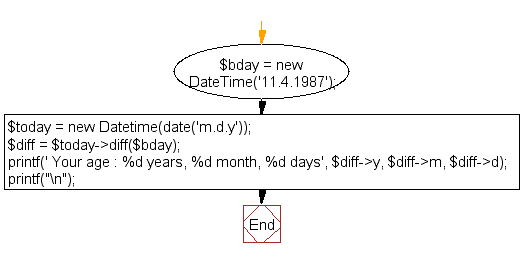
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP function to get start and end date of a week (by week number) of a particular year.
Next: Write a PHP script to calculate weeks between two dates.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.