PHP Exercises: Memoize a given function results in memory
Write a PHP program to memoize a given function results in memory.
Note: In computing, memoization or memoisation is an optimization technique used primarily to speed up computer programs by storing the results of expensive function calls and returning the cached result when the same inputs occur again.
Sample Solution:
PHP Code:
<?php
// Licence: https://bit.ly/2CFA5XY
// Function definition for 'memoize' that takes a function as a parameter
function memoize($func)
{
// Use an anonymous function to create a closure that captures and persists a static cache variable
return function () use ($func) {
// Static cache variable to store memoized results
static $cache = [];
// Get the arguments passed to the memoized function
$args = func_get_args();
// Serialize the arguments to create a cache key
$key = serialize($args);
// Flag to indicate whether the result is retrieved from the cache or calculated
$cached = true;
// Check if the result for the current arguments is already in the cache
if (!isset($cache[$key])) {
// If not in the cache, calculate the result using the original function and store it in the cache
$cache[$key] = $func(...$args);
$cached = false;
}
// Return an associative array containing the result and a boolean indicating whether it was cached
return ['result' => $cache[$key], 'cached' => $cached];
};
}
// Create a memoized version of the 'add 10' function using 'memoize'
$memoizedAdd = memoize(
function ($num) {
return $num + 10;
}
);
// Call the memoized function with an argument and display the result and cache status using 'var_dump'
var_dump($memoizedAdd(5));
// Call the memoized function with another argument and display the result and cache status using 'var_dump'
var_dump($memoizedAdd(6));
// Call the memoized function with the same argument as before and display the result and cache status using 'var_dump'
var_dump($memoizedAdd(5));
?>
Explanation:
- Function Definition:
- The memoize function is defined to take another function ($func) as a parameter.
- Anonymous Function:
- Inside memoize, an anonymous function (closure) is returned that captures $func. This allows the memoization logic to be applied to any function passed to memoize.
- Static Cache Variable:
- A static variable $cache is declared within the anonymous function to persist cached results across calls. It will store previously computed results for specific argument combinations.
- Argument Handling:
- func_get_args() is used to retrieve the arguments passed to the memoized function, which are stored in the $args array.
- Cache Key Generation:
- The arguments are serialized to create a unique cache key using serialize($args). This key allows the function to identify if the result for these arguments has already been computed and cached.
- Cache Lookup:
- The code checks if the result for the current arguments exists in the cache (isset($cache[$key])):
- If it exists, the cached result is returned with a cached flag set to true.
- If it does not exist, the original function is called to compute the result, which is then stored in the cache, and the cached flag is set to false.
- Return Value:
- The memoized function returns an associative array containing the computed result and a boolean cached indicating whether the result was retrieved from the cache.
- Creating a Memoized Function:
- A memoized version of a simple function that adds 10 to its input is created and stored in the variable $memoizedAdd.
- Function Calls and Output:
- The memoized function is called with the argument 5, and the result along with the cache status is displayed using var_dump. This will compute 15 and mark it as not cached.
- The memoized function is called again with the argument 6, computing 16 and marking it as not cached.
- Finally, the memoized function is called again with the argument 5, which retrieves the result from the cache, resulting in 15 and marking it as cached.
Output:
array(2) { ["result"]=> int(15) ["cached"]=> bool(false) } array(2) { ["result"]=> int(16) ["cached"]=> bool(false) } array(2) { ["result"]=> int(15) ["cached"]=> bool(true) }
Flowchart:
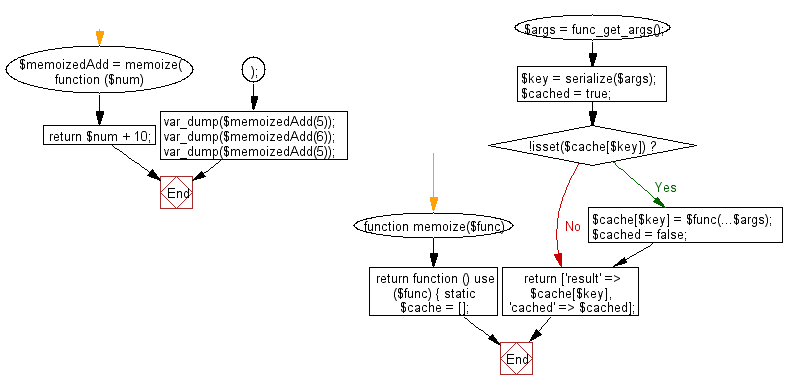
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous:Write a PHP program to create a new function that composes multiple functions into a single callable.
Next: Write a PHP program to curry a function to take arguments in multiple calls.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics