PHP Exercises: Create a new function that composes multiple functions into a single callable
98. Compose Multiple Functions into One
Write a PHP program to create a new function that composes multiple functions into a single callable.
Sample Solution:
PHP Code:
<?php
// Licence: https://bit.ly/2CFA5XY
// Function definition for 'compose' that takes a variable number of functions as parameters
function compose(...$functions)
{
// Use 'array_reduce' to iterate through the functions and create a composition
return array_reduce(
$functions,
function ($carry, $function) {
// Return a new function that applies the current function to the result of the previous function
return function ($x) use ($carry, $function) {
return $function($carry($x));
};
},
// The initial function that simply returns the input
function ($x) {
return $x;
}
);
}
// Create a composition of two functions: add 2 and multiply by 4
$compose = compose(
// add 2
function ($x) {
return $x + 2;
},
// multiply by 4
function ($x) {
return $x * 4;
}
);
// Call the composed function with an argument and display the result using 'print_r'
print_r($compose(2));
// Display a newline
echo("\n");
// Call the composed function with another argument and display the result using 'print_r'
print_r($compose(3));
?>
Explanation:
- Function Definition:
- The compose function is defined to take a variable number of functions as parameters using the splat operator (...$functions).
- Function Composition:
- It uses array_reduce to iterate over the provided functions and build a composition of them.
- The callback function takes two parameters: $carry (the accumulated result) and $function (the current function being processed).
- Returning a New Function:
- The inner function takes an argument $x and applies the current function to the result of the previous composed function ($carry($x)).
- The initial function passed to array_reduce simply returns its input, acting as the base case for composition.
- Creating a Composed Function:
- The $compose variable stores a new function that adds 2 and then multiplies the result by 4.
- This is done by passing two anonymous functions to compose:
- The first function adds 2 to the input.
- The second function multiplies the result by 4.
- Function Calls and Output:
- The composed function is called with the argument 2, and the result is displayed using print_r, which evaluates to 16 (i.e., (2 + 2) * 4).
- A newline is printed.
- The composed function is called again with the argument 3, and the result is displayed, which evaluates to 20 (i.e., (3 + 2) * 4).
Output:
16 20
Flowchart:
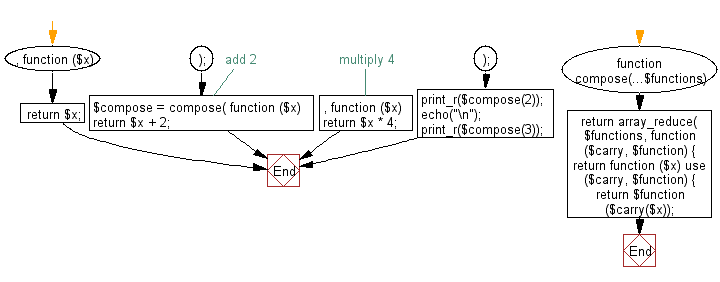
For more Practice: Solve these Related Problems:
- Write a PHP script to create a function composer that chains multiple functions into a single callable.
- Write a PHP function to take an array of functions and return a new function that applies them sequentially.
- Write a PHP script to implement function composition so that the output of one function is fed as the input to the next.
- Write a PHP script to build a composite function from several callables using higher-order programming techniques.
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous:Write a PHP program to decapitalize the first letter of the string and then adds it with rest of the string.
Next: Write a PHP program to memoize a given function results in memory.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.